v-modal 将string int 转 int
时间: 2023-06-22 09:21:41 浏览: 56
您好,根据您的问题,我理解您想要实现的功能是:将 Vue 组件中的字符串类型转换为整数类型,并将 v-model 绑定的值转换为整数类型。
在 Vue 组件中,v-model 绑定的值可以是字符串类型或整数类型,所以可以直接将字符串类型的值转换为整数类型。可以使用 JavaScript 中的 parseInt() 函数来实现这一转换。例如:
```
<template>
<div>
<input v-model="strValue">
<p>{{ intValue }}</p>
</div>
</template>
<script>
export default {
data() {
return {
strValue: '123'
}
},
computed: {
intValue: {
get() {
return parseInt(this.strValue)
},
set(value) {
this.strValue = value.toString()
}
}
}
}
</script>
```
在上面的代码中,我们使用了 computed 计算属性来实现字符串类型和整数类型的双向绑定。其中,getIntValue() 函数用于将字符串类型的值转换为整数类型的值,setIntValue() 函数用于将整数类型的值转换为字符串类型的值。
希望这个回答能够帮到您!
相关问题
@GetMapping("/add_rooms") public String addRoom(@RequestParam("roomname") String roomName, @RequestParam("roomaddress") String roomAddress, @RequestParam("roomtype") String roomType, @RequestParam("roomfood") String roomFood, @RequestParam("roomwifi") int roomWifi, @RequestParam("roomprice") Float roomPrice) { Room room = new Room(); room.setR_name(roomName); room.setR_type(roomType); room.setR_food(roomFood); room.setR_wifi(roomWifi); room.setR_price(roomPrice); if (roomService.addRoom(room)) { System.out.println("成功"); } else { System.out.println("失败"); } return "roomAdd"; }添加成功如何在页面提示成功
在页面中提示添加成功,你可以使用以下方法之一:
1. 在HTML页面中添加一个成功提示的元素,例如一个 `<div>`,并设置其内容为成功信息。可以使用JavaScript来控制该元素的显示和隐藏。
```html
<div id="successMessage" style="display: none;">添加成功!</div>
```
在JavaScript中,你可以根据添加成功与否来控制该元素的显示和隐藏:
```javascript
if (response === "成功") {
document.getElementById("successMessage").style.display = "block";
} else {
document.getElementById("successMessage").style.display = "none";
}
```
2. 使用模态框(Modal)或弹出框(Alert)来显示成功信息。你可以使用Bootstrap等前端框架来实现这些组件。
```html
<!-- 使用Bootstrap的Modal组件 -->
<div class="modal fade" id="successModal" tabindex="-1" role="dialog" aria-labelledby="successModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="successModalLabel">成功</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
添加成功!
</div>
</div>
</div>
</div>
```
在JavaScript中,你可以通过调用相关函数来显示模态框:
```javascript
if (response === "成功") {
$('#successModal').modal('show');
} else {
// 处理失败情况
}
```
以上是两种常见的在页面中提示添加成功的方法,你可以根据自己的需求选择其中一种来实现。
我使用 MySQL 创建了一个用户表TbUser,userId的类型为Integer,并且使用@GetMapping("/alterListUsers") public String alterListUsers(@RequestParam(defaultValue = "1") int pageNum, Model model) { Page<TbUser> page = tbUserService.findUsers(pageNum); List<TbUser> userList = page.getContent(); model.addAttribute("userList", userList); model.addAttribute("currentPage", pageNum); model.addAttribute("totalPages", page.getTotalPages()); return "management/user-alter"; }完成了用户分页查询功能,查询的结果返回了user-alter.html,请使用springboot+hibernate+thymeleaf帮助我完成编辑用户的功能,点击编辑按钮后,弹窗一个表单可以修改用户所有信息,点击确定后将信息保存到数据库,包含DAO、Controller、Entity、Service、impl的详细代码
好的,下面是对应的代码:
TbUser Entity类:
```java
@Entity
@Table(name = "tb_user")
public class TbUser {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "user_id")
private Integer userId;
@Column(name = "user_name")
private String userName;
@Column(name = "user_password")
private String userPassword;
// 省略getter和setter方法
}
```
TbUserRepository接口:
```java
@Repository
public interface TbUserRepository extends JpaRepository<TbUser, Integer> {
}
```
TbUserService接口:
```java
public interface TbUserService {
Page<TbUser> findUsers(int pageNum);
TbUser updateUser(TbUser user);
}
```
TbUserServiceImpl类:
```java
@Service
public class TbUserServiceImpl implements TbUserService {
@Autowired
private TbUserRepository userRepository;
@Override
public Page<TbUser> findUsers(int pageNum) {
Pageable pageable = PageRequest.of(pageNum - 1, 10);
return userRepository.findAll(pageable);
}
@Override
public TbUser updateUser(TbUser user) {
return userRepository.save(user);
}
}
```
UserController类:
```java
@Controller
@RequestMapping("/user")
public class UserController {
@Autowired
private TbUserService userService;
@GetMapping("/alterListUsers")
public String alterListUsers(@RequestParam(defaultValue = "1") int pageNum, Model model) {
Page<TbUser> page = userService.findUsers(pageNum);
List<TbUser> userList = page.getContent();
model.addAttribute("userList", userList);
model.addAttribute("currentPage", pageNum);
model.addAttribute("totalPages", page.getTotalPages());
return "management/user-alter";
}
@PostMapping("/updateUser")
@ResponseBody
public TbUser updateUser(@RequestBody TbUser user) {
return userService.updateUser(user);
}
}
```
user-alter.html页面:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>User Alter</title>
</head>
<body>
<div th:each="user : ${userList}">
<div>
<span th:text="${user.userId}"></span>
<span th:text="${user.userName}"></span>
<span th:text="${user.userPassword}"></span>
<button onclick="editUser(this)" th:data-userId="${user.userId}"
th:data-userName="${user.userName}" th:data-userPassword="${user.userPassword}">Edit
</button>
</div>
</div>
<!-- 弹窗表单 -->
<div id="editUserDialog" style="display: none">
<form id="editUserForm">
<input type="hidden" name="userId" id="editUserId">
User Name: <input type="text" name="userName" id="editUserName"><br>
Password: <input type="password" name="userPassword" id="editUserPassword"><br>
<button type="submit">Submit</button>
</form>
</div>
<!-- 引入jQuery和Ajax -->
<script th:src="@{/webjars/jquery/jquery.min.js}"></script>
<script th:src="@{/webjars/bootstrap/js/bootstrap.min.js}"></script>
<script>
function editUser(button) {
var userId = $(button).data("userid");
var userName = $(button).data("username");
var userPassword = $(button).data("userpassword");
$("#editUserId").val(userId);
$("#editUserName").val(userName);
$("#editUserPassword").val(userPassword);
// 弹出表单
$("#editUserDialog").dialog({
modal: true,
width: 400,
buttons: {
"Submit": function () {
var userId = $("#editUserId").val();
var userName = $("#editUserName").val();
var userPassword = $("#editUserPassword").val();
// 发送Ajax请求保存用户信息
$.ajax({
url: "/user/updateUser",
type: "POST",
contentType: "application/json",
data: JSON.stringify({
userId: userId,
userName: userName,
userPassword: userPassword
}),
success: function (data) {
// 更新页面信息
$(button).data("username", userName);
$(button).data("userpassword", userPassword);
$(button).siblings("span").eq(1).text(userName);
$(button).siblings("span").eq(2).text(userPassword);
alert("Update user successfully!");
$("#editUserDialog").dialog("close");
},
error: function () {
alert("Update user failed!");
}
});
},
"Cancel": function () {
$("#editUserDialog").dialog("close");
}
}
});
}
</script>
</body>
</html>
```
注:需要在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.webjars</groupId>
<artifactId>jquery</artifactId>
<version>3.6.0</version>
</dependency>
<dependency>
<groupId>org.webjars</groupId>
<artifactId>bootstrap</artifactId>
<version>5.0.2</version>
</dependency>
```
相关推荐
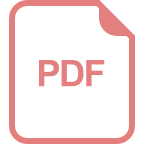
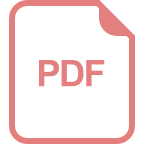













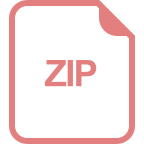