compare_exchange_strong例子、
时间: 2023-05-26 15:06:23 浏览: 163
`compare_exchange_strong` 是 C++ 中一个原子操作,可以对给定的 atomic 对象执行比较和交换操作。它的基本形式如下:
```
bool compare_exchange_strong(T& expected, T desired, memory_order order = memory_order_seq_cst) volatile noexcept;
```
这个函数的作用是比较 atomic 对象的当前值和 `expected` 是否相等。如果相等,就将对象的值设置为 `desired`。这个操作是原子的,因为它在完成之前会进行加锁和解锁。如果相等,返回 `true`,否则返回 `false`。
下面是一个简单的例子,展示了如何使用 `compare_exchange_strong` 来实现线程安全的计数器:
```c++
#include <atomic>
#include <iostream>
#include <thread>
std::atomic<int> counter(0);
void increment() {
for (int i = 0; i < 100; ++i) {
int expected = counter.load();
while (!counter.compare_exchange_strong(expected, expected + 1)) {
expected = counter.load();
}
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Final counter value is " << counter << "\n";
return 0;
}
```
在这个例子中,我们定义了一个计数器 atomic 对象 `counter`,并创建了两个线程,每个线程都会对计数器增加 100 次。
在 `increment` 函数内部,我们首先使用 `load` 函数获取当前计数器的值。然后使用 `compare_exchange_strong` 函数不断地尝试将当前值加 1,直到操作成功为止。在这个过程中,我们使用一个 while 循环来不断重试,直到操作成功为止。
这个例子展示了如何使用 `compare_exchange_strong` 函数来实现线程安全的计数器,避免了多个线程同时访问共享变量产生的竞态条件。
相关推荐
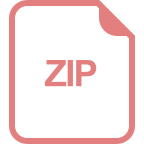
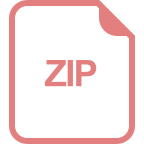
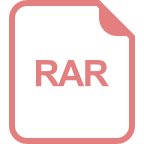














