请写一个OC瀑布流列表
时间: 2023-12-13 15:02:28 浏览: 211

瀑布流的实例
以下是一个简单的OC瀑布流列表的实现:
首先,我们需要导入UICollectionView和UICollectionViewFlowLayout:
```
#import <UIKit/UIKit.h>
@interface WaterfallViewController : UIViewController<UICollectionViewDataSource, UICollectionViewDelegate>
@end
```
接下来,在ViewController中实现UICollectionViewDataSource和UICollectionViewDelegate协议:
```
@interface WaterfallViewController ()<UICollectionViewDataSource, UICollectionViewDelegate>
@property (nonatomic, strong) UICollectionView *collectionView;
@property (nonatomic, strong) NSMutableArray *dataList;
@end
@implementation WaterfallViewController
- (void)viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor whiteColor];
[self.view addSubview:self.collectionView];
[self loadData];
}
- (void)loadData {
// 模拟数据
self.dataList = [NSMutableArray arrayWithObjects:@(200), @(150), @(180), @(220), @(250), @(190), @(170), @(240), @(160), @(200), @(150), @(180), @(220), @(250), @(190), @(170), @(240), @(160), nil];
}
#pragma mark - UICollectionViewDataSource
- (NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section {
return self.dataList.count;
}
- (UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath {
static NSString *cellIdentifier = @"cellIdentifier";
UICollectionViewCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:cellIdentifier forIndexPath:indexPath];
cell.backgroundColor = [UIColor grayColor];
// 设置高度
CGFloat height = [self.dataList[indexPath.row] floatValue];
cell.frame = CGRectMake(cell.frame.origin.x, cell.frame.origin.y, cell.frame.size.width, height);
return cell;
}
#pragma mark - UICollectionViewDelegate
- (void)collectionView:(UICollectionView *)collectionView didSelectItemAtIndexPath:(NSIndexPath *)indexPath {
NSLog(@"Selected item at index %ld", (long)indexPath.row);
}
#pragma mark - Lazy Load
- (UICollectionView *)collectionView {
if (!_collectionView) {
UICollectionViewFlowLayout *layout = [[UICollectionViewFlowLayout alloc] init];
layout.minimumLineSpacing = 10;
layout.minimumInteritemSpacing = 10;
layout.sectionInset = UIEdgeInsetsMake(10, 10, 10, 10);
CGFloat width = (self.view.frame.size.width - 30) / 2;
layout.itemSize = CGSizeMake(width, 0);
_collectionView = [[UICollectionView alloc] initWithFrame:self.view.bounds collectionViewLayout:layout];
_collectionView.backgroundColor = [UIColor whiteColor];
_collectionView.dataSource = self;
_collectionView.delegate = self;
[_collectionView registerClass:[UICollectionViewCell class] forCellWithReuseIdentifier:@"cellIdentifier"];
}
return _collectionView;
}
@end
```
这里我们模拟了一个简单的数据源数组dataList,包含了一些高度数据。在UICollectionViewDataSource代理方法中,我们根据对应的高度数据设置了每个cell的高度,实现了瀑布流效果。
另外,我们通过UICollectionViewFlowLayout设置了UICollectionView的布局方式,包括行间距、列间距、边距和每个cell的大小等。
最后,我们将UICollectionView注册到了ViewController中,并在viewDidLoad方法中加载数据源和UICollectionView。
阅读全文
相关推荐
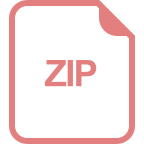

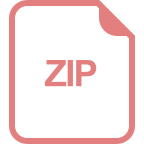
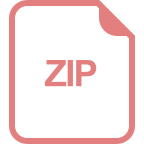
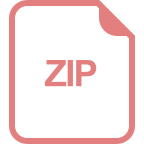
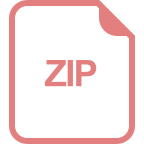
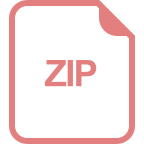
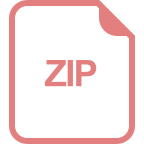
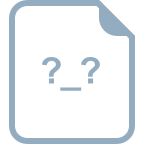
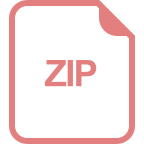
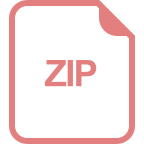
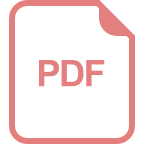

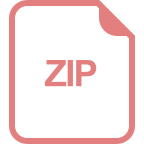