numpy写逻辑回归预测的代码
时间: 2023-06-29 16:16:55 浏览: 39
下面是一个简单的使用 NumPy 实现逻辑回归预测的代码示例:
```python
import numpy as np
# 生成训练数据
X = np.array([[1, 2], [2, 3], [3, 4], [4, 5], [5, 6]])
y = np.array([0, 0, 1, 1, 1])
# 初始化参数
theta = np.zeros((X.shape[1] + 1, 1))
X = np.hstack((np.ones((X.shape[0], 1)), X))
# 定义 sigmoid 函数
def sigmoid(z):
return 1 / (1 + np.exp(-z))
# 定义代价函数
def cost_function(X, y, theta):
m = y.size
h = sigmoid(X @ theta)
J = -1 / m * (y @ np.log(h) + (1 - y) @ np.log(1 - h))
return J
# 定义梯度下降函数
def gradient_descent(X, y, theta, alpha, num_iters):
m = y.size
J_history = np.zeros(num_iters)
for i in range(num_iters):
h = sigmoid(X @ theta)
theta -= alpha * 1 / m * X.T @ (h - y)
J_history[i] = cost_function(X, y, theta)
return theta, J_history
# 进行训练
theta, J_history = gradient_descent(X, y, theta, 0.1, 100)
# 预测新数据
X_new = np.array([[6, 7], [7, 8]])
X_new = np.hstack((np.ones((X_new.shape[0], 1)), X_new))
y_pred = sigmoid(X_new @ theta)
print(y_pred)
```
在这个示例中,我们先生成了一些训练数据,然后使用梯度下降法对模型进行训练,最后对新数据进行预测。请注意,这里的代价函数和梯度下降函数都是使用了向量化的 NumPy 实现,这使得代码变得更加简洁和高效。
相关推荐
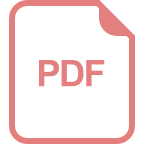
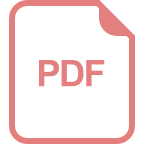
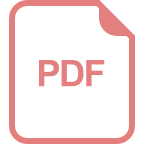














