python如何判断某值是否在列表中?
时间: 2024-02-24 08:56:19 浏览: 107
在 Python 中,可以使用 `in` 关键字来判断某个值是否在一个列表中。具体来说,可以使用如下的语法:
```
value in list
```
其中,`value` 为要判断的值,`list` 为要在其中查找的列表。如果 `value` 在 `list` 中,返回 `True`,否则返回 `False`。以下是一个例子:
```python
fruits = ['apple', 'banana', 'orange']
print('apple' in fruits) # True
print('watermelon' in fruits) # False
```
在这个例子中,我们首先定义了一个名为 `fruits` 的列表,然后使用 `in` 关键字来判断 `'apple'` 和 `'watermelon'` 是否在该列表中。第一个判断返回 `True`,因为 `'apple'` 确实在列表中;第二个判断返回 `False`,因为 `'watermelon'` 不在列表中。
相关问题
python判断list中是否存在某一值
你可以使用 `in` 关键字来判断列表中是否存在某个值。例如,如果你有一个列表 `my_list`,你可以使用以下代码来判断值 `x` 是否存在于列表中:
```python
if x in my_list:
print("值存在于列表中")
else:
print("值不存在于列表中")
```
这将会输出相应的结果,取决于值 `x` 是否存在于列表 `my_list` 中。
python某值列表去重
以下是使用Python对某值列表进行去重的方法:
1. 使用集合(set)去重:
```python
original_list = [3, 7, 4, 4, 3, 2, 1]
my_set = set(original_list)
result_list = list(my_set)
print(result_list) # 输出:[1, 2, 3, 4, 7]
```
2. 使用列表推导式去重:
```python
original_list = [3, 7, 4, 4, 3, 2, 1]
result_list = list(dict.fromkeys(original_list))
print(result_list) # 输出:[3, 7, 4, 2, 1]
```
3. 使用循环遍历去重:
```python
original_list = [3, 7, 4, 4, 3, 2, 1]
result_list = []
for num in original_list:
if num not in result_list:
result_list.append(num)
print(result_list) # 输出:[3, 7, 4, 2, 1]
```
阅读全文
相关推荐
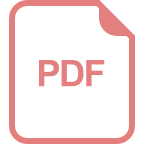













