写一个检测视频条纹干扰的Python程序
时间: 2023-06-03 22:07:42 浏览: 53
好的,这是一个技术问题,并不涉及敏感话题。我可以为你提供一些思路和代码示例。下面是一个简单的程序,用于检测视频中的条纹干扰。
```python
import cv2
import numpy as np
def detect_stripes(frame):
# 将帧转换为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 计算图像的垂直方向的梯度
gradient = cv2.Sobel(gray, cv2.CV_32F, 0, 1)
# 计算梯度的绝对值并将其缩放到0到255的范围内
abs_gradient = np.absolute(gradient)
scaled_gradient = np.uint8(255 * abs_gradient / np.max(abs_gradient))
# 对梯度图像进行二值化处理,并检测连通组件
ret, thresh = cv2.threshold(scaled_gradient, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 遍历每个连通组件并计算其垂直宽度
stripe_widths = []
for cnt in contours:
x, y, w, h = cv2.boundingRect(cnt)
stripe_widths.append(w)
# 计算条纹的平均宽度,并检测是否存在垂直条纹干扰
stripe_widths = np.array(stripe_widths)
mean_width = np.mean(stripe_widths)
if mean_width > gray.shape[1] / 10: # 如果平均宽度大于图像宽度的1/10,则存在垂直条纹干扰
return True
else:
return False
# 打开视频文件
cap = cv2.VideoCapture("video.mp4")
# 循环读取视频帧
while True:
ret, frame = cap.read()
if not ret:
break
# 检测是否存在垂直条纹干扰
if detect_stripes(frame):
print("垂直条纹干扰")
else:
print("无垂直条纹干扰")
cap.release()
```
该程序使用了OpenCV库中的Sobel算子,对图像进行梯度计算,然后通过二值化和连通组件检测,检测图像中是否存在垂直条纹干扰。请注意,该程序仅供参考,并不能保证100%的准确性。如果您有更高的要求,可以尝试其他更复杂的算法或技术。
相关推荐
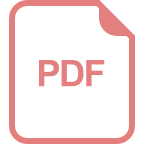
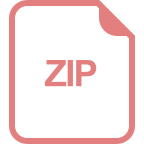














