用c语言写算术表达式求值。演示程序和源程序已给出。用户从键盘输入一个算术表达式,数字都是十进制整型(必须支持负数),运算符支持+-*/还有()。 编写源程序中的 double deal_exp (char *exp) 函数,对这个算术表达式求值,返回结果。要求用两个栈,一个储存数字,一个储存运算符
时间: 2024-02-03 14:11:19 浏览: 41
下面是一个用C语言实现的算术表达式求值程序,使用了两个栈来处理运算符和数字:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_EXPR_LENGTH 100
typedef struct {
int data[MAX_EXPR_LENGTH];
int top;
} int_stack;
typedef struct {
char data[MAX_EXPR_LENGTH];
int top;
} char_stack;
void init_int_stack(int_stack* s) {
s->top = -1;
}
void init_char_stack(char_stack* s) {
s->top = -1;
}
int is_int_stack_empty(int_stack* s) {
return s->top == -1;
}
int is_char_stack_empty(char_stack* s) {
return s->top == -1;
}
int is_int_stack_full(int_stack* s) {
return s->top == MAX_EXPR_LENGTH - 1;
}
int is_char_stack_full(char_stack* s) {
return s->top == MAX_EXPR_LENGTH - 1;
}
void push_int(int_stack* s, int x) {
if (is_int_stack_full(s)) {
printf("Error: stack full\n");
exit(1);
}
s->data[++s->top] = x;
}
void push_char(char_stack* s, char c) {
if (is_char_stack_full(s)) {
printf("Error: stack full\n");
exit(1);
}
s->data[++s->top] = c;
}
int pop_int(int_stack* s) {
if (is_int_stack_empty(s)) {
printf("Error: stack empty\n");
exit(1);
}
return s->data[s->top--];
}
char pop_char(char_stack* s) {
if (is_char_stack_empty(s)) {
printf("Error: stack empty\n");
exit(1);
}
return s->data[s->top--];
}
int peek_int(int_stack* s) {
if (is_int_stack_empty(s)) {
printf("Error: stack empty\n");
exit(1);
}
return s->data[s->top];
}
char peek_char(char_stack* s) {
if (is_char_stack_empty(s)) {
printf("Error: stack empty\n");
exit(1);
}
return s->data[s->top];
}
int is_digit(char c) {
return c >= '0' && c <= '9';
}
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
int precedence(char c) {
switch (c) {
case '+': return 1;
case '-': return 1;
case '*': return 2;
case '/': return 2;
default: return 0;
}
}
double deal_exp(char* exp) {
int i, j, len, op1, op2;
char c;
double num, result;
int_stack num_stack;
char_stack op_stack;
init_int_stack(&num_stack);
init_char_stack(&op_stack);
len = strlen(exp);
for (i = 0; i < len; i++) {
if (exp[i] == ' ') {
continue;
} else if (is_digit(exp[i])) {
j = i;
while (j < len && is_digit(exp[j])) {
j++;
}
num = atof(exp + i);
i = j - 1;
push_int(&num_stack, num);
} else if (exp[i] == '(') {
push_char(&op_stack, exp[i]);
} else if (exp[i] == ')') {
while (!is_char_stack_empty(&op_stack) && peek_char(&op_stack) != '(') {
op2 = pop_int(&num_stack);
op1 = pop_int(&num_stack);
c = pop_char(&op_stack);
if (c == '+') {
push_int(&num_stack, op1 + op2);
} else if (c == '-') {
push_int(&num_stack, op1 - op2);
} else if (c == '*') {
push_int(&num_stack, op1 * op2);
} else if (c == '/') {
push_int(&num_stack, op1 / op2);
}
}
pop_char(&op_stack);
} else if (is_operator(exp[i])) {
while (!is_char_stack_empty(&op_stack) && precedence(peek_char(&op_stack)) >= precedence(exp[i])) {
op2 = pop_int(&num_stack);
op1 = pop_int(&num_stack);
c = pop_char(&op_stack);
if (c == '+') {
push_int(&num_stack, op1 + op2);
} else if (c == '-') {
push_int(&num_stack, op1 - op2);
} else if (c == '*') {
push_int(&num_stack, op1 * op2);
} else if (c == '/') {
push_int(&num_stack, op1 / op2);
}
}
push_char(&op_stack, exp[i]);
} else {
printf("Error: invalid input\n");
exit(1);
}
}
while (!is_char_stack_empty(&op_stack)) {
op2 = pop_int(&num_stack);
op1 = pop_int(&num_stack);
c = pop_char(&op_stack);
if (c == '+') {
push_int(&num_stack, op1 + op2);
} else if (c == '-') {
push_int(&num_stack, op1 - op2);
} else if (c == '*') {
push_int(&num_stack, op1 * op2);
} else if (c == '/') {
push_int(&num_stack, op1 / op2);
}
}
result = pop_int(&num_stack);
if (!is_int_stack_empty(&num_stack) || !is_char_stack_empty(&op_stack)) {
printf("Error: invalid input\n");
exit(1);
}
return result;
}
int main() {
char expr[MAX_EXPR_LENGTH];
printf("Enter an arithmetic expression: ");
fgets(expr, MAX_EXPR_LENGTH, stdin);
printf("Result: %.2f\n", deal_exp(expr));
return 0;
}
```
这个程序使用了两个栈,一个存储数字,一个存储运算符。在处理表达式时,程序从左到右依次遍历每个字符,如果遇到数字,就将它解析成一个整数并压入数字栈;如果遇到左括号,就将它压入运算符栈;如果遇到右括号,就将运算符栈中的运算符弹出并计算,直到遇到左括号为止;如果遇到运算符,就将它与运算符栈中的运算符比较优先级,如果它的优先级不高于栈顶运算符的优先级,就将栈顶运算符弹出并计算,直到栈顶运算符的优先级低于它为止,然后将它压入运算符栈。最后,将数字栈中的结果弹出即为表达式的值。
相关推荐
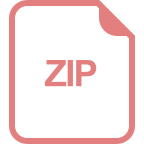
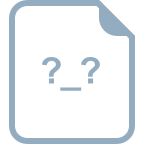












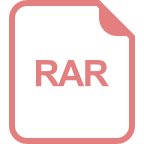