java 给出一组无序整数,求出中位数,如果求最中间两个数的平均数,向下取整即可(不需要使用浮点数)
时间: 2023-05-30 12:03:41 浏览: 151
可以使用快速选择算法来解决这个问题。快速选择算法类似于快速排序算法,但是它只需要找到第k小的元素,而不需要对整个数组进行排序。
具体实现步骤如下:
1. 随机选择数组中一个元素作为基准值pivot。
2. 将数组分为两部分:小于pivot的元素组成的子数组和大于等于pivot的元素组成的子数组。
3. 如果小于pivot的元素的个数大于等于k,则在小于pivot的元素组成的子数组中递归查找第k小的元素。
4. 如果小于pivot的元素的个数小于k,则在大于等于pivot的元素组成的子数组中递归查找第k - 小于pivot的元素个数 - 1小的元素。
5. 重复以上步骤,直到找到第k小的元素。
Java代码如下:
```
public static int findMedian(int[] nums) {
int n = nums.length;
if (n % 2 == 1) {
// 如果数组长度为奇数,中位数是第(n + 1) / 2小的数
return quickSelect(nums, 0, n - 1, (n + 1) / 2);
} else {
// 如果数组长度为偶数,中位数是第n / 2小的数和第(n / 2 + 1)小的数的平均数
return (quickSelect(nums, 0, n - 1, n / 2) + quickSelect(nums, 0, n - 1, n / 2 + 1)) / 2;
}
}
private static int quickSelect(int[] nums, int left, int right, int k) {
if (left == right) {
return nums[left];
}
int pivotIndex = partition(nums, left, right);
int count = pivotIndex - left + 1;
if (k == count) {
return nums[pivotIndex];
} else if (k < count) {
return quickSelect(nums, left, pivotIndex - 1, k);
} else {
return quickSelect(nums, pivotIndex + 1, right, k - count);
}
}
private static int partition(int[] nums, int left, int right) {
int pivotIndex = left + (int) (Math.random() * (right - left + 1));
int pivot = nums[pivotIndex];
swap(nums, pivotIndex, right);
int i = left;
for (int j = left; j < right; j++) {
if (nums[j] < pivot) {
swap(nums, i, j);
i++;
}
}
swap(nums, i, right);
return i;
}
private static void swap(int[] nums, int i, int j) {
int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
```
阅读全文
相关推荐
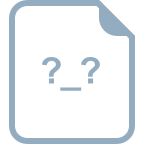
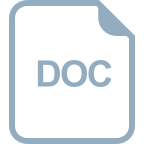
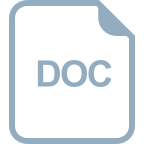
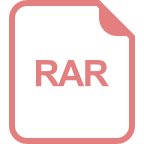
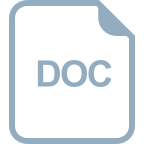
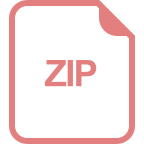
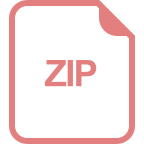
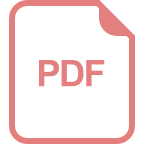
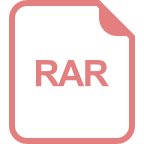
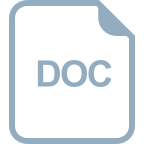
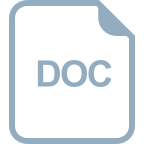
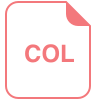
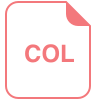


