public class ThreadHomework { public static void main(String[] args) { T1 t1 = new T1(); T2 t2 = new T2(); Thread th1 = new Thread(t1); Thread th2 = new Thread(t2); th1.start(); th2.start(); } } class T1 implements Runnable { @Override public void run() { for (int i = 1; i <= 10; i++) { System.out.println("hello,world"); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } } class T2 implements Runnable { @Override public void run() { for (int i = 1; i <= 5; i++) { System.out.println("hi"); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } } 修改代码使其输出为hi 1 hello,world 1 hi 2 hello,world 2 hi 3 hello,world 3 hi 4 hello,world 4 hi 5 hello,world 5 hello,world 6 hello,world 7 hello,world 8 hello,world 9 hello,world 10
时间: 2024-01-12 18:05:45 浏览: 29
以下是修改后的Java程序代码:
```
public class ThreadHomework {
public static void main(String[] args) {
T1 t1 = new T1();
T2 t2 = new T2(t1);
Thread th1 = new Thread(t1);
Thread th2 = new Thread(t2);
th1.start();
th2.start();
}
}
class T1 implements Runnable {
@Override
public void run() {
for (int i = 1; i <= 10; i++) {
synchronized (this) {
System.out.println("hello,world " + i);
notify();
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
synchronized (this) {
notify();
}
}
}
class T2 implements Runnable {
private T1 t1;
public T2(T1 t1) {
this.t1 = t1;
}
@Override
public void run() {
for (int i = 1; i <= 5; i++) {
synchronized (t1) {
try {
t1.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("hi " + i);
t1.notify();
}
}
}
}
```
在上面的代码中,我们对T1和T2类进行了修改,以实现按照指定的输出顺序输出。具体修改如下:
- 在T1类中,我们使用synchronized关键字对输出语句进行了加锁,并使用wait()和notify()方法来控制线程的执行顺序。在每次输出后,我们调用notify()方法唤醒T2线程并让T1线程进入等待状态,直到T2线程输出完毕后再唤醒T1线程继续执行。
- 在T2类中,我们添加了一个T1类型的成员变量t1,并在构造方法中初始化。在run()方法中,我们使用synchronized关键字对t1对象进行加锁,并使用wait()和notify()方法来控制线程的执行顺序。在每次输出后,我们调用notify()方法唤醒T1线程并让T2线程进入等待状态,直到T1线程输出完毕后再唤醒T2线程继续执行。
当程序运行时,将会按照指定的顺序输出“hi”和“hello,world”,直到输出完毕后两个线程将会自动退出。
相关推荐
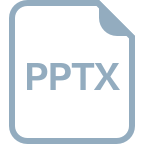
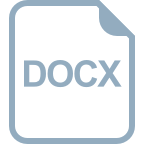
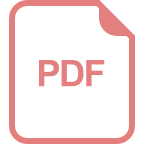
















