python俄罗斯方块
时间: 2023-06-23 16:28:53 浏览: 132
Python 语言可以使用 Pygame 或者 Pygame Zero 库来开发俄罗斯方块游戏。Pygame 是一个专门用于游戏开发的 Python 库,而 Pygame Zero 则是基于 Pygame 开发的简化版库,更加适合初学者使用。
以下是一个简单的 Pygame Zero 实现的俄罗斯方块游戏代码示例:
```python
import random
import pgzrun
# 定义游戏窗口大小
WIDTH = 200
HEIGHT = 400
# 定义俄罗斯方块的各种形状
shapes = [
[[1, 1],
[1, 1]],
[[1, 1, 1],
[0, 1, 0]],
[[1, 1, 1],
[1, 0, 0]],
[[1, 1, 0],
[0, 1, 1]],
[[0, 1, 1],
[1, 1, 0]],
[[0, 1, 0],
[1, 1, 1]],
[[1, 0, 0],
[1, 1, 1]]
]
# 定义俄罗斯方块的颜色值
colors = [
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255),
(255, 255, 255)
]
# 定义俄罗斯方块类
class Tetromino:
def __init__(self):
self.x = WIDTH // 2 - 1
self.y = 0
self.shape = random.choice(shapes)
self.color = random.choice(colors)
def move_down(self):
self.y += 1
def move_left(self):
self.x -= 1
def move_right(self):
self.x += 1
def rotate(self):
self.shape = list(zip(*self.shape[::-1]))
def draw(self):
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j]:
rect = Rect((self.x + j) * 20, (self.y + i) * 20, 20, 20)
screen.draw.filled_rect(rect, self.color)
def check_collision(self, board):
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j]:
if self.y + i >= len(board) or \
self.x + j < 0 or self.x + j >= len(board[0]) or \
board[self.y + i][self.x + j]:
return True
return False
def add_to_board(self, board):
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j]:
board[self.y + i][self.x + j] = self.color
# 定义游戏状态
game_state = 0
board = [[(0, 0, 0) for _ in range(10)] for _ in range(20)]
tetromino = Tetromino()
# 定义游戏循环
def update():
global game_state, board, tetromino
if game_state == 0:
# 移动俄罗斯方块
tetromino.move_down()
# 检查是否碰撞
if tetromino.check_collision(board):
tetromino.move_up()
tetromino.add_to_board(board)
tetromino = Tetromino()
if tetromino.check_collision(board):
game_state = 1
# 检查是否满行
for i in range(len(board)):
if all(board[i]):
board.pop(i)
board.insert(0, [(0, 0, 0) for _ in range(10)])
def draw():
screen.clear()
# 绘制俄罗斯方块
tetromino.draw()
# 绘制游戏区域
for i in range(len(board)):
for j in range(len(board[i])):
rect = Rect(j * 20, i * 20, 20, 20)
screen.draw.filled_rect(rect, board[i][j])
# 启动游戏循环
pgzrun.go()
```
这段代码实现了一个基本的俄罗斯方块游戏,可以在 Pygame Zero 中运行。在游戏中,方块会从上往下掉落,玩家可以左右移动方块,让它落在合适的位置,当方块堆满一行时,该行会消失,得分增加。当方块堆满整个游戏区域时,游戏结束。
阅读全文
相关推荐
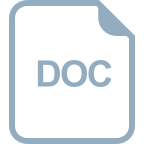
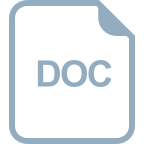
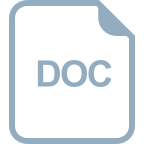

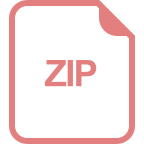





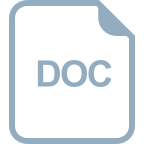
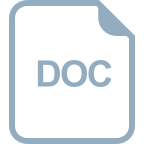
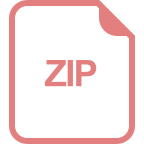
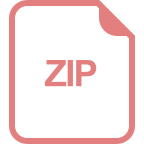
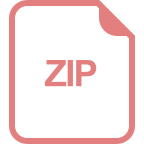