用python在一个文件后面追加另一个文件中的内容
时间: 2024-02-28 13:52:04 浏览: 19
好的,您可以使用Python中的文件操作来实现这个需求。以下是示例代码:
```python
with open('file1.txt', 'a') as f1, open('file2.txt', 'r') as f2:
content = f2.read()
f1.write(content)
```
这段代码会打开两个文件,file1.txt和file2.txt。然后它会读取file2.txt中的所有内容,将其追加到file1.txt中,最后关闭两个文件。您可以根据需要修改文件名和路径。
相关问题
python在一个文件后面追加另一个文件中的内容。
可以使用Python的文件操作来实现将一个文件的内容追加到另一个文件的末尾。具体步骤如下:
1. 打开要追加的文件和要被追加的文件。
```
file_to_append = open("file_to_append.txt", "r")
file_to_be_appended = open("file_to_be_appended.txt", "a")
```
2. 读取要追加的文件的内容。
```
content = file_to_append.read()
```
3. 将读取的内容写入要被追加的文件。
```
file_to_be_appended.write(content)
```
4. 关闭文件。
```
file_to_append.close()
file_to_be_appended.close()
```
完整代码如下:
```
file_to_append = open("file_to_append.txt", "r")
file_to_be_appended = open("file_to_be_appended.txt", "a")
content = file_to_append.read()
file_to_be_appended.write(content)
file_to_append.close()
file_to_be_appended.close()
```
python在文件后面追加内容
可以使用open函数的mode参数中的'a'来以追加模式打开文件,然后向文件中写入内容。示例代码如下:
```python
with open('file.txt', 'a') as f:
f.write('new content\n')
```
上面的代码会打开名为file.txt的文件,如果该文件存在,则以追加模式打开;如果不存在,则会创建该文件。然后将字符串'new content\n'写入文件末尾。注意,'\n'是换行符,可以根据需要增加或删除。最后,with语句块结束时,文件会自动关闭。
相关推荐
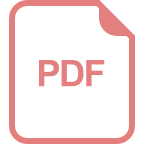
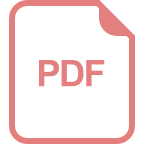












