用c++代码在Windows桌面应用程序中以各种字体格式输出文本:字体样式、字体大小等。
时间: 2024-02-25 08:56:29 浏览: 24
以下是一个例子,演示如何在 Windows 桌面应用程序中以不同的字体格式输出文本。这个例子使用了 Win32 API 来实现。
```c++
#include <Windows.h>
LRESULT CALLBACK WndProc(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
const char szClassName[] = "MyWindowClass";
WNDCLASSEX wc;
HWND hWnd;
MSG msg;
// Register the window class
wc.cbSize = sizeof(WNDCLASSEX);
wc.style = 0;
wc.lpfnWndProc = WndProc;
wc.cbClsExtra = 0;
wc.cbWndExtra = 0;
wc.hInstance = hInstance;
wc.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.lpszMenuName = NULL;
wc.lpszClassName = szClassName;
wc.hIconSm = LoadIcon(NULL, IDI_APPLICATION);
if (!RegisterClassEx(&wc))
{
MessageBox(NULL, "Window Registration Failed!", "Error!", MB_ICONEXCLAMATION | MB_OK);
return 0;
}
// Create the window
hWnd = CreateWindowEx(
WS_EX_CLIENTEDGE,
szClassName,
"My Window",
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, 240, 120,
NULL, NULL, hInstance, NULL);
if (hWnd == NULL)
{
MessageBox(NULL, "Window Creation Failed!", "Error!", MB_ICONEXCLAMATION | MB_OK);
return 0;
}
// Show the window
ShowWindow(hWnd, nCmdShow);
UpdateWindow(hWnd);
// Run the message loop
while (GetMessage(&msg, NULL, 0, 0) > 0)
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch (msg)
{
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hWnd, &ps);
HFONT hFont;
LOGFONT lf;
// Set up the font
lf.lfHeight = 24;
lf.lfWidth = 0;
lf.lfEscapement = 0;
lf.lfOrientation = 0;
lf.lfWeight = FW_NORMAL;
lf.lfItalic = TRUE;
lf.lfUnderline = FALSE;
lf.lfStrikeOut = FALSE;
lf.lfCharSet = DEFAULT_CHARSET;
lf.lfOutPrecision = OUT_DEFAULT_PRECIS;
lf.lfClipPrecision = CLIP_DEFAULT_PRECIS;
lf.lfQuality = DEFAULT_QUALITY;
lf.lfPitchAndFamily = DEFAULT_PITCH | FF_DONTCARE;
strcpy_s(lf.lfFaceName, "Courier New");
// Create the font
hFont = CreateFontIndirect(&lf);
// Select the font into the device context
HFONT hOldFont = (HFONT)SelectObject(hdc, hFont);
// Draw some text with the font
TextOut(hdc, 10, 10, "Hello, World!", 13);
// Change the font size
lf.lfHeight = 36;
DeleteObject(hFont);
hFont = CreateFontIndirect(&lf);
SelectObject(hdc, hFont);
TextOut(hdc, 10, 50, "Hello, World!", 13);
// Clean up
SelectObject(hdc, hOldFont);
DeleteObject(hFont);
EndPaint(hWnd, &ps);
}
return 0;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
default:
return DefWindowProc(hWnd, msg, wParam, lParam);
}
}
```
这个例子创建了一个窗口,并使用了 `CreateFontIndirect` 函数创建了一个字体。字体的样式和大小都可以通过修改 LOGFONT 结构体的成员来设置。在 WM_PAINT 消息中,使用 `SelectObject` 函数将字体选入设备上下文,使用 `TextOut` 函数在窗口中绘制了一些文本。这个例子只是演示了如何在 Windows 桌面应用程序中输出文本,你可以根据自己的需求修改字体和文本内容。
相关推荐
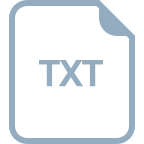
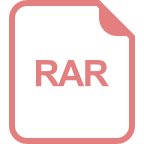
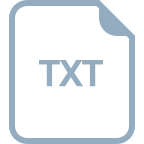














