用java模拟一个龟兔赛跑的应用程序,要求打印窗体模拟龟兔赛跑
时间: 2023-07-16 10:13:27 浏览: 113
好的,以下是一个简单的Java程序来模拟龟兔赛跑:
```java
import javax.swing.*;
import java.awt.*;
public class RacingGame extends JFrame {
private JLabel lblTortoise;
private JLabel lblHare;
public RacingGame() {
setTitle("龟兔赛跑");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(600, 200);
setResizable(false);
// 创建两个标签来表示乌龟和兔子
lblTortoise = new JLabel(new ImageIcon("tortoise.png"));
lblHare = new JLabel(new ImageIcon("hare.png"));
// 将标签添加到窗体中
JPanel contentPane = new JPanel();
contentPane.setLayout(null);
contentPane.add(lblTortoise);
contentPane.add(lblHare);
setContentPane(contentPane);
// 设置标签的位置和大小
lblTortoise.setBounds(0, 50, 100, 100);
lblHare.setBounds(0, 0, 100, 100);
setVisible(true);
// 开始比赛
startRace();
}
private void startRace() {
int tortoisePos = 0;
int harePos = 0;
while (true) {
// 计算乌龟和兔子的下一步位置
tortoisePos += (int)(Math.random() * 3) + 1;
harePos += (int)(Math.random() * 6) + 1;
// 更新标签的位置
lblTortoise.setLocation(tortoisePos, 50);
lblHare.setLocation(harePos, 0);
// 判断比赛是否结束
if (tortoisePos >= 500) {
JOptionPane.showMessageDialog(this, "乌龟赢了!");
break;
}
else if (harePos >= 500) {
JOptionPane.showMessageDialog(this, "兔子赢了!");
break;
}
// 暂停一下,让用户看清楚每步的移动
try {
Thread.sleep(100);
}
catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
new RacingGame();
}
}
```
在这个程序中,我们使用了Java Swing库来创建窗体和标签,并使用了一个循环来模拟比赛的过程。每次循环中,我们使用Math.random()方法来计算乌龟和兔子的下一步位置,然后更新标签的位置。最后,我们通过判断乌龟和兔子是否到达终点来结束比赛。整个程序比较简单,适合初学者练手。
阅读全文
相关推荐

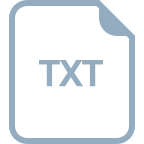


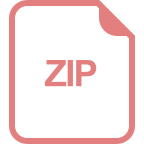
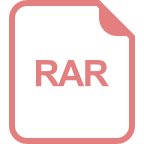





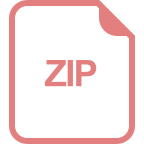