编写一个模拟龟兔赛跑的窗体应用程序,绘制不同颜色的两个圆分别代表乌龟和兔子,设置一个按钮,单击按键后,龟兔开始赛跑。
时间: 2023-07-16 12:15:38 浏览: 46
以下是一个简单的模拟龟兔赛跑的窗体应用程序,使用 C# 语言和 Windows Forms 库绘制不同颜色的两个圆分别代表乌龟和兔子,并设置一个按钮,单击按键后,龟兔开始赛跑:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
namespace TurtleAndRabbitRace
{
public partial class Form1 : Form
{
private const int RACE_TRACK_LENGTH = 500;
private const int RACE_TRACK_WIDTH = 100;
private const int TURTLE_START_POS = 0;
private const int RABBIT_START_POS = 0;
private const int TURTLE_SPEED = 10;
private const int RABBIT_SPEED = 20;
private const int FINISH_LINE = RACE_TRACK_LENGTH - RACE_TRACK_WIDTH;
private readonly Random random = new Random();
private int turtlePos = TURTLE_START_POS;
private int rabbitPos = RABBIT_START_POS;
public Form1()
{
InitializeComponent();
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
Graphics graphics = e.Graphics;
// Draw the race track
Pen pen = new Pen(Color.Black, RACE_TRACK_WIDTH);
graphics.DrawLine(pen, 0, ClientSize.Height / 2, ClientSize.Width, ClientSize.Height / 2);
// Draw the turtle and rabbit
Brush turtleBrush = new SolidBrush(Color.Green);
Brush rabbitBrush = new SolidBrush(Color.Red);
graphics.FillEllipse(turtleBrush, turtlePos, ClientSize.Height / 2 - RACE_TRACK_WIDTH / 2, RACE_TRACK_WIDTH, RACE_TRACK_WIDTH);
graphics.FillEllipse(rabbitBrush, rabbitPos, ClientSize.Height / 2 - RACE_TRACK_WIDTH / 2 - RACE_TRACK_WIDTH, RACE_TRACK_WIDTH, RACE_TRACK_WIDTH);
}
private void button1_Click(object sender, EventArgs e)
{
timer1.Start();
}
private void timer1_Tick(object sender, EventArgs e)
{
// Move the turtle and rabbit randomly
turtlePos += TURTLE_SPEED;
rabbitPos += RABBIT_SPEED * random.Next(2);
// Check if they have reached the finish line
if (turtlePos >= FINISH_LINE || rabbitPos >= FINISH_LINE)
{
timer1.Stop();
if (turtlePos > rabbitPos)
{
MessageBox.Show("Turtle wins!");
}
else if (rabbitPos > turtlePos)
{
MessageBox.Show("Rabbit wins!");
}
else
{
MessageBox.Show("It's a tie!");
}
// Reset the positions
turtlePos = TURTLE_START_POS;
rabbitPos = RABBIT_START_POS;
}
Invalidate();
}
}
}
```
在窗体上添加一个按钮和一个 Timer 控件,并将 Timer 控件的 Interval 属性设置为 100,然后将上述代码复制到窗体类中即可。
当单击按钮时,程序将启动 Timer 控件,并每 100 毫秒重绘窗体以更新乌龟和兔子的位置。每次 Tick 事件发生时,程序将随机移动乌龟和兔子,并检查它们是否已经到达终点线。如果是,则停止计时器,并显示获胜者。最后,程序将重置乌龟和兔子的位置,以便下一次比赛。
相关推荐
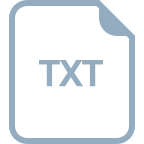
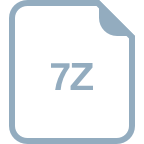














