C语言 有十个学生,学号是101-110,共有十节课,首先编写一个结构体数组记录10名学生的学号,以及10门课的成绩,这些成绩都是一个50-100的随机数。编写一个函数,通过10门课的总成绩排序这个数组,排序完成后,回到main函数,打印排序后的10个学生信息
时间: 2023-10-27 16:05:52 浏览: 79
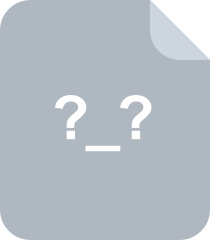
编程实现:(1) 键入某组学生(共 10 人)的学号、姓名、组成原理考试 成绩、数据结构考试成绩、汇编语言考试成绩
好的,以下是实现该功能的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define NUM_STUDENTS 10
#define NUM_COURSES 10
struct student {
int id;
int scores[NUM_COURSES];
int total_score;
};
void generate_scores(struct student *s) {
int i;
for (i = 0; i < NUM_COURSES; i++) {
s->scores[i] = rand() % 51 + 50; // 50-100的随机数
s->total_score += s->scores[i];
}
}
void sort_students(struct student *students) {
int i, j;
struct student temp;
for (i = 0; i < NUM_STUDENTS - 1; i++) {
for (j = i + 1; j < NUM_STUDENTS; j++) {
if (students[i].total_score < students[j].total_score) {
temp = students[i];
students[i] = students[j];
students[j] = temp;
}
}
}
}
void print_students(struct student *students) {
int i, j;
for (i = 0; i < NUM_STUDENTS; i++) {
printf("学号:%d,总成绩:%d,各科成绩:", students[i].id, students[i].total_score);
for (j = 0; j < NUM_COURSES; j++) {
printf("%d ", students[i].scores[j]);
}
printf("\n");
}
}
int main() {
struct student students[NUM_STUDENTS];
int i;
// 初始化随机数生成器
srand(time(NULL));
// 初始化学生信息
for (i = 0; i < NUM_STUDENTS; i++) {
students[i].id = 101 + i;
generate_scores(&students[i]);
}
// 排序学生信息
sort_students(students);
// 打印学生信息
print_students(students);
return 0;
}
```
该程序首先定义了一个名为`student`的结构体,用于记录每个学生的学号、各科成绩以及总成绩。接着,通过`generate_scores`函数随机生成每个学生的成绩,并计算出总成绩。然后,通过`sort_students`函数将学生数组按照总成绩排序。最后,通过`print_students`函数打印排序后的学生信息。
注意,该程序使用了`rand`函数生成随机数,因此需要先调用`srand`函数初始化随机数生成器。在本例中,我们使用了当前时间作为随机数生成器的种子,以保证每次运行程序时得到的随机数序列都不同。
阅读全文
相关推荐
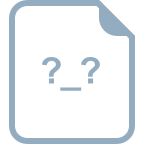














