帮我写一个消除文法左递归的c++代码
时间: 2023-08-20 12:33:02 浏览: 53
当一个文法存在左递归时,我们可以通过以下步骤消除它:
1. 对于每个非终结符A,将所有以A开头的产生式移动到A的后面,并将A替换为一个新的非终结符A'。
2. 对于每个以A开头的产生式,将其左边的A替换为A'。
下面是一个简单的C++代码来消除文法左递归:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// 消除非终结符A的左递归
void eliminate_left_recursion(string A, vector<string> &productions) {
vector<string> alpha, beta;
for (string production : productions) {
if (production[0] == A) {
alpha.push_back(production.substr(1));
} else {
beta.push_back(production);
}
}
if (!alpha.empty()) {
string A_prime = A + "'";
cout << A << " -> ";
for (string b : beta) {
cout << b << A_prime << " | ";
}
cout << endl << A_prime << " -> ";
for (string a : alpha) {
if (a[0] == A) {
cout << a.substr(1) << A_prime << " | ";
} else {
cout << a << A_prime << " | ";
}
}
cout << "epsilon" << endl;
vector<string> new_productions = {A_prime + " -> epsilon"};
eliminate_left_recursion(A_prime, alpha);
for (string b : beta) {
if (b[0] != A) {
new_productions.push_back(b + A_prime);
}
}
productions = new_productions;
}
}
int main() {
vector<string> productions = {"A -> Ab | c"};
eliminate_left_recursion('A', productions);
for (string production : productions) {
cout << production << endl;
}
return 0;
}
```
在这个例子中,我们的文法只有一个非终结符A和两个产生式Ab和c。我们调用`eliminate_left_recursion`函数来消除A的左递归,并打印出新的产生式。输出为:
```
A -> cA'
A' -> AbA' | epsilon
```
相关推荐
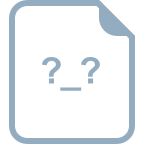














