#include<stdio.h> #include<sys/types.h> #include<unistd.h> #include<stdlib.h> int main(int argc, char const *argv[]) { int a = 20; pid_t x = vfork(); if(x==0) { a+=20; printf("%d\n", getpid()); printf("%d\n", a); printf("%p",&a); } if(x>0) { a+=1; printf("%d\n", getpid()); printf("%d\n", a); printf("%p",&a); } return 0; }
时间: 2024-04-21 21:29:20 浏览: 146
这是一个使用vfork()函数创建子进程的C语言程序,程序中定义了一个整型变量a,并且在子进程和父进程中对a进行了不同的操作,最后输出了进程ID和变量a的值和地址。
vfork()函数与fork()函数类似,都可以用于创建子进程,但vfork()函数的创建效率比fork()高,因为它不会复制父进程的地址空间,而是与父进程共享地址空间。但是vfork()函数的使用比较危险,因为子进程会在父进程的地址空间中运行,如果子进程修改了父进程的变量或者执行了一些不安全的操作,就会导致程序崩溃或者出现不可预料的错误。
在程序中,首先定义了一个整型变量a,并赋初值为20。然后调用vfork()函数创建子进程,如果子进程创建成功,就会执行if语句中的代码块,这里将a的值增加了20,并输出了进程ID、变量a的值和地址。接着判断当前进程是否为父进程,如果是父进程,则将a的值增加1,并输出进程ID、变量a的值和地址。最后程序结束,返回0。
需要注意的是,程序中对变量a的修改会影响到父进程和子进程中的变量a,因为它们共享同一个地址空间。此外,程序中输出的变量a的地址是相同的,因为它们指向的是同一个变量。
相关问题
#include <stdio.h> #include <sys/types.h> #include <unistd.h> #include<stdlib.h> int main () { int pid;int fd[2];char S[20];pipe (fd) ;pid=fork (); if(0==pid){ int i=0 ; for (;i<10; i++){ sprintf(S,"Hello,No %d ",i);write(fd[1],S,20) ; sleep (1);} exit (0) ; } else { int i=0 ; for ( ;i<10; i++){ read (fd[0],S,20);printf ("%s",S);sleep (1);} exit (0) ; } }
这是一个用 C 语言写的管道通信程序。程序中使用了系统调用函数 pipe、fork、read、write、sleep 和 exit。
程序的功能是创建一个管道,然后通过 fork 函数创建一个子进程。子进程会向管道中写入 10 条字符串,每隔 1 秒写入一次。父进程则从管道中读取数据,并打印出来,也是每隔 1 秒读取一次。
需要注意的是,管道是一种半双工的通信方式,所以程序中创建了两个文件描述符:fd[0] 用于读取管道数据,fd[1] 用于写入管道数据。同时,父子进程中都需要关闭不需要的文件描述符,以避免资源浪费和出现意外情况。
#include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> #include <unistd.h> #include <fcntl.h> #include <pthread.h> #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> #include <ctype.h> #include <sys/pps.h> #include <sys/types.h> #include "camera.h" #include <encoder.h> #include "ppsFunc.h" #include "timeDiff.h"
这是一段C语言的代码,使用了一些系统库和自定义头文件。其中包括了网络编程相关的库,如sys/socket.h和netinet/in.h,以及多线程相关的库pthread.h。另外还有一些自定义的头文件,如camera.h和encoder.h,可能是该程序所需的一些外部依赖。该程序可能是一个视频流的采集和编码程序,同时还使用了PPS和时间差等功能。
阅读全文
相关推荐
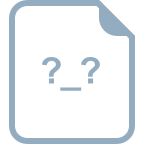















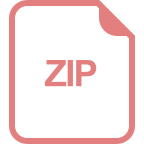