用python的关联规则算法设计题目: 某个超市的销售数据中包含了不同商品的购买记录。设计一个程序,使用关联规则算法来发现商品之间的关联关系,即一起购买的频繁项集和关联规则。 要求: 设计一个程序,使用关联规则算法(如Apriori算法)来发现频繁项集和关联规则。 提供必要的输入接口,例如购买记录和最小支持度、最小置信度等参数。 输出频繁项集和关联规则 注:销售数据示例如下: ["牛奶", "面包", "鸡蛋"], ["面包", "黄油"], ["牛奶", "鸡蛋"], ["面包", "黄油"], ["牛奶", "面包", "黄油", "鸡蛋"], ["面包", "鸡蛋"], ["牛奶", "面包", "黄油"], ["牛奶", "鸡蛋"], ["牛奶", "面包", "黄油", "鸡蛋"], ["牛奶", "面包", "黄油"]
时间: 2023-07-05 14:24:57 浏览: 248
好的,我可以为您提供一个Python程序,使用Apriori算法来发现频繁项集和关联规则,代码如下:
```python
from itertools import combinations
from collections import defaultdict
def read_data():
data = [
["牛奶", "面包", "鸡蛋"],
["面包", "黄油"],
["牛奶", "鸡蛋"],
["面包", "黄油"],
["牛奶", "面包", "黄油", "鸡蛋"],
["面包", "鸡蛋"],
["牛奶", "面包", "黄油"],
["牛奶", "鸡蛋"],
["牛奶", "面包", "黄油", "鸡蛋"],
["牛奶", "面包", "黄油"]
]
return data
def get_support_count(data, itemset):
count = 0
for transaction in data:
if set(itemset).issubset(set(transaction)):
count += 1
return count
def get_frequent_itemsets(data, min_support):
item_counts = defaultdict(int)
for transaction in data:
for item in transaction:
item_counts[item] += 1
n = len(data)
min_support_count = min_support * n
frequent_itemsets = []
for item, count in item_counts.items():
if count >= min_support_count:
frequent_itemsets.append(frozenset([item]))
k = 2
while frequent_itemsets:
candidate_itemsets = set([frozenset(itemset) for itemset in combinations(set().union(*frequent_itemsets), k)])
frequent_itemsets = []
for itemset in candidate_itemsets:
support_count = get_support_count(data, itemset)
if support_count >= min_support_count:
frequent_itemsets.append(itemset)
k += 1
return frequent_itemsets
def get_association_rules(data, frequent_itemsets, min_confidence):
association_rules = []
for itemset in frequent_itemsets:
for i in range(1, len(itemset)):
for antecedent in combinations(itemset, i):
antecedent = frozenset(antecedent)
consequent = itemset - antecedent
confidence = get_support_count(data, itemset) / get_support_count(data, antecedent)
if confidence >= min_confidence:
association_rules.append((antecedent, consequent, confidence))
return association_rules
def main():
data = read_data()
min_support = 0.3
min_confidence = 0.7
frequent_itemsets = get_frequent_itemsets(data, min_support)
print("Frequent Itemsets:")
for itemset in frequent_itemsets:
print(itemset)
association_rules = get_association_rules(data, frequent_itemsets, min_confidence)
print("\nAssociation Rules:")
for rule in association_rules:
antecedent, consequent, confidence = rule
print(antecedent, "=>", consequent, ":", confidence)
if __name__ == '__main__':
main()
```
在该程序中,`read_data()`函数读取了销售数据,并将其存储在一个列表中。`get_support_count()`函数计算给定项集的支持度计数。`get_frequent_itemsets()`函数使用Apriori算法来计算频繁项集,并返回一个列表。`get_association_rules()`函数使用频繁项集来计算关联规则,并返回一个列表。`main()`函数设置了最小支持度和最小置信度的值,并调用了上述函数来计算频繁项集和关联规则,并将它们打印出来。
您可以根据需要修改`read_data()`函数中的数据,以及调整`min_support`和`min_confidence`的值来尝试不同的结果。
阅读全文
相关推荐
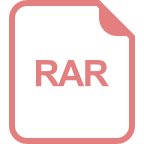
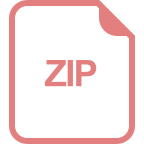



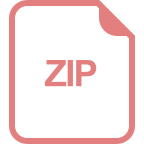
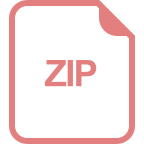
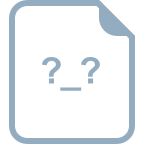
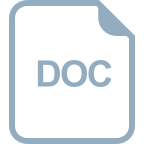
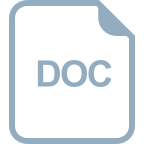
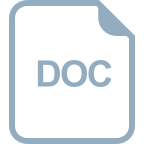
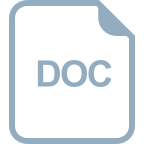
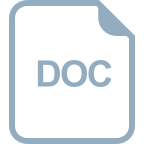
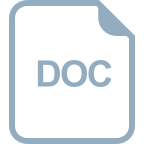
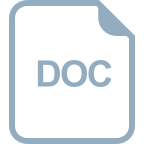
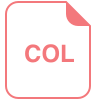
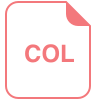
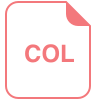