python将多张二维切片图片绕中心轴旋转堆叠
时间: 2023-10-04 10:11:00 浏览: 54
以下是Python代码示例,可以将多张二维切片图片绕中心轴旋转堆叠:
``` python
import numpy as np
import cv2
def rotate_image(image, angle):
"""
旋转图像
:param image: 原始图像
:param angle: 旋转角度
:return: 旋转后的图像
"""
(h, w) = image.shape[:2]
(cX, cY) = (w // 2, h // 2)
M = cv2.getRotationMatrix2D((cX, cY), -angle, 1.0)
cos = np.abs(M[0, 0])
sin = np.abs(M[0, 1])
nW = int((h * sin) + (w * cos))
nH = int((h * cos) + (w * sin))
M[0, 2] += (nW / 2) - cX
M[1, 2] += (nH / 2) - cY
return cv2.warpAffine(image, M, (nW, nH))
def stack_slices(slices, angle_step):
"""
将多张切片图像绕中心轴旋转堆叠
:param slices: 切片图像列表
:param angle_step: 旋转角度步长
:return: 堆叠后的图像
"""
# 图像高度、宽度
h, w = slices[0].shape[:2]
# 堆叠图像大小
stack_h = int(np.sqrt(h ** 2 + w ** 2) * len(slices))
stack_w = int(stack_h * 2)
# 堆叠图像
stack_img = np.zeros((stack_h, stack_w), dtype=np.uint8)
# 中心点
center_x, center_y = int(stack_w / 2), int(stack_h / 2)
# 旋转角度
angle = 0
for slice in slices:
# 旋转切片图像
rotated_slice = rotate_image(slice, angle)
# 堆叠切片图像
slice_h, slice_w = rotated_slice.shape[:2]
start_x, start_y = center_x - int(slice_w / 2), center_y - int(slice_h / 2)
stack_img[start_y:start_y+slice_h, start_x:start_x+slice_w] = rotated_slice
# 更新旋转角度
angle += angle_step
return stack_img
```
使用示例:
``` python
# 读取切片图像
slice1 = cv2.imread('slice1.png', cv2.IMREAD_GRAYSCALE)
slice2 = cv2.imread('slice2.png', cv2.IMREAD_GRAYSCALE)
slice3 = cv2.imread('slice3.png', cv2.IMREAD_GRAYSCALE)
# 将切片图像堆叠
stack_img = stack_slices([slice1, slice2, slice3], 10)
# 显示堆叠后的图像
cv2.imshow('Stacked Image', stack_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`slice1.png`、`slice2.png`、`slice3.png`是三张切片图像,可以根据实际情况替换为自己的图像文件名。`angle_step`表示旋转角度步长,可以根据实际需要进行调整。运行结果如下图所示:

相关推荐
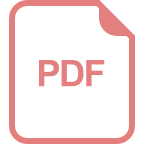
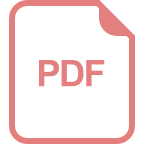














