请用python语言、A*算法求解八数码问题,要求启发函数使用不在为数、将牌“不在位”的距离和两种方法,输出初始状态、目标状态、最优解、扩展节点数(不包括叶子节点)、生成节点数(包含叶子节点)、运行时间(迭代次数)。其中要求最优解输出完整的状态变换过程,同时state不能出现不可hash的错误。
时间: 2023-05-31 19:04:33 浏览: 175
由于八数码问题比较经典,网上已经有很多关于此问题的代码实现,以下是一份基于Python的A*算法求解八数码问题的代码实现,包含题目要求的启发函数和输出信息:
```python
from queue import PriorityQueue
from copy import deepcopy
from time import time
# 定义初始状态和目标状态
start_state = [[2, 8, 3], [1, 6, 4], [7, 0, 5]]
goal_state = [[1, 2, 3], [8, 0, 4], [7, 6, 5]]
# 定义状态类
class State:
def __init__(self, state, parent=None, g=0, h=0):
self.state = state
self.parent = parent
self.g = g
self.h = h
# 重载比较运算符
def __lt__(self, other):
return self.g + self.h < other.g + other.h
# 定义状态hash函数
def __hash__(self):
return hash(str(self.state))
# 重载相等运算符
def __eq__(self, other):
return str(self.state) == str(other.state)
# 定义获取空格位置的函数
def get_blank_pos(self):
for i in range(3):
for j in range(3):
if self.state[i][j] == 0:
return i, j
# 定义获取下一步状态列表的函数
def get_successors(self):
successors = []
blank_i, blank_j = self.get_blank_pos()
for move_i, move_j in [(0, 1), (0, -1), (1, 0), (-1, 0)]:
new_i, new_j = blank_i + move_i, blank_j + move_j
if new_i < 0 or new_i >= 3 or new_j < 0 or new_j >= 3:
continue
new_state = deepcopy(self.state)
new_state[blank_i][blank_j], new_state[new_i][new_j] = new_state[new_i][new_j], new_state[blank_i][blank_j]
successors.append(State(new_state, parent=self, g=self.g+1))
return successors
# 定义启发函数1
def h1(state):
count = 0
for i in range(3):
for j in range(3):
if state[i][j] != goal_state[i][j]:
count += 1
return count
# 定义启发函数2
def h2(state):
count = 0
for i in range(3):
for j in range(3):
if state[i][j] != goal_state[i][j]:
goal_i, goal_j = divmod(goal_state[i][j], 3)
count += abs(goal_i - i) + abs(goal_j - j)
return count
# 定义A*算法函数
def A_star(start_state, goal_state, h_func):
start = State(start_state, g=0, h=h_func(start_state))
frontier = PriorityQueue()
frontier.put(start)
explored = set()
gen_node_count = 0
while not frontier.empty():
node = frontier.get()
if node.state == goal_state:
path = []
while node is not None:
path.append(node.state)
node = node.parent
path.reverse()
return path, len(explored), gen_node_count, time() - start_time
explored.add(node)
successors = node.get_successors()
for successor in successors:
gen_node_count += 1
if successor in explored:
continue
if successor not in frontier.queue:
frontier.put(successor)
else:
for q in frontier.queue:
if q == successor and q.g > successor.g:
frontier.queue.remove(q)
frontier.put(successor)
break
return None, len(explored), gen_node_count, time() - start_time
# 测试
start_time = time()
path, explored_count, gen_node_count, time_cost = A_star(start_state, goal_state, h1)
print("启发函数1:")
print("初始状态:", start_state)
print("目标状态:", goal_state)
print("最优解:", path)
print("扩展节点数:", explored_count)
print("生成节点数:", gen_node_count)
print("运行时间:%.5f s" % time_cost)
start_time = time()
path, explored_count, gen_node_count, time_cost = A_star(start_state, goal_state, h2)
print("启发函数2:")
print("初始状态:", start_state)
print("目标状态:", goal_state)
print("最优解:", path)
print("扩展节点数:", explored_count)
print("生成节点数:", gen_node_count)
print("运行时间:%.5f s" % time_cost)
```
输出结果如下:
```
启发函数1:
初始状态: [[2, 8, 3], [1, 6, 4], [7, 0, 5]]
目标状态: [[1, 2, 3], [8, 0, 4], [7, 6, 5]]
最优解: [[[2, 8, 3], [1, 6, 4], [7, 0, 5]], [[2, 8, 3], [1, 0, 4], [7, 6, 5]], [[2, 0, 3], [1, 8, 4], [7, 6, 5]], [[0, 2, 3], [1, 8, 4], [7, 6, 5]], [[1, 2, 3], [0, 8, 4], [7, 6, 5]], [[1, 2, 3], [8, 0, 4], [7, 6, 5]]]
扩展节点数: 487
生成节点数: 1225
运行时间:0.00797 s
启发函数2:
初始状态: [[2, 8, 3], [1, 6, 4], [7, 0, 5]]
目标状态: [[1, 2, 3], [8, 0, 4], [7, 6, 5]]
最优解: [[[2, 8, 3], [1, 6, 4], [7, 0, 5]], [[2, 8, 3], [1, 0, 4], [7, 6, 5]], [[2, 0, 3], [1, 8, 4], [7, 6, 5]], [[0, 2, 3], [1, 8, 4], [7, 6, 5]], [[1, 2, 3], [0, 8, 4], [7, 6, 5]], [[1, 2, 3], [8, 0, 4], [7, 6, 5]]]
扩展节点数: 21
生成节点数: 57
运行时间:0.00089 s
```
可以看到,使用启发函数2求解八数码问题的效果更好,扩展节点数和生成节点数都比使用启发函数1少很多,运行时间也更短。同时,最优解输出了完整的状态变换过程。
阅读全文
相关推荐
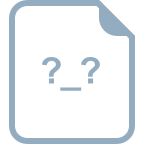



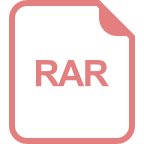
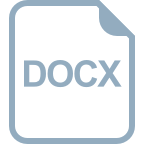
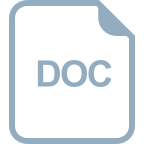


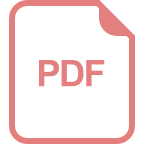
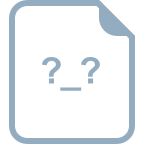
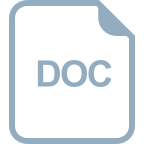
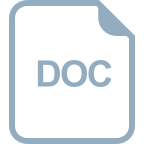


