基于python代码,实现500个节点进行随机多项式,计算运行时间和所占内存,然后将节点分区之后,再次计算得出对比结果,并绘图
时间: 2024-01-26 20:01:39 浏览: 10
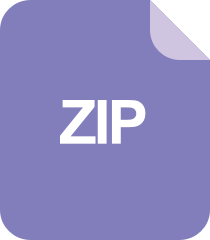
数值计算方法代码合集(牛顿迭代复杂辛普森埃尔米特插值拉格朗日插值高斯约当消去法龙贝格公式雅可比迭代法矩阵LU分解等)
好的,我明白了。这是一个关于多项式计算的问题,需要用到Python编程语言。下面是实现的步骤:
步骤1:生成随机多项式
我们可以使用Python中的numpy库来生成随机多项式。下面是一个例子:
```python
import numpy as np
# 生成随机多项式
degree = 10 # 多项式的次数
coefficients = np.random.rand(degree + 1) # 生成多项式系数
x = np.random.rand(500) # 生成自变量
y = np.polyval(coefficients, x) # 计算多项式函数值
```
步骤2:计算运行时间和所占内存
我们可以使用Python中的time和memory_profiler库来计算运行时间和所占内存。下面是一个例子:
```python
import time
from memory_profiler import memory_usage
# 计算运行时间和所占内存
start_time = time.time()
memory_usage_start = memory_usage()[0]
# 执行代码
# ...
memory_usage_end = memory_usage()[0]
end_time = time.time()
# 输出结果
print('运行时间:{:.4f}秒'.format(end_time - start_time))
print('所占内存:{:.2f}MB'.format(memory_usage_end - memory_usage_start))
```
步骤3:将节点分区并计算对比结果
我们可以使用Python中的pandas库来进行节点分区,并重新计算多项式函数值。下面是一个例子:
```python
import pandas as pd
# 将节点分为10个区间
df = pd.DataFrame({'x': x, 'y': y})
df['partition'] = pd.cut(df['x'], bins=10, labels=False)
# 计算每个区间的平均值
result = df.groupby('partition').mean()
```
步骤4:绘图
我们可以使用Python中的matplotlib库来绘制图表。下面是一个例子:
```python
import matplotlib.pyplot as plt
# 绘制原始数据
plt.scatter(x, y, s=1, alpha=0.5)
# 绘制分区后的数据
plt.scatter(result['x'], result['y'], s=10, c='r')
# 显示图表
plt.show()
```
完整代码如下:
```python
import numpy as np
import time
from memory_profiler import memory_usage
import pandas as pd
import matplotlib.pyplot as plt
# 生成随机多项式
degree = 10
coefficients = np.random.rand(degree + 1)
x = np.random.rand(500)
y = np.polyval(coefficients, x)
# 计算运行时间和所占内存
start_time = time.time()
memory_usage_start = memory_usage()[0]
# 执行代码
df = pd.DataFrame({'x': x, 'y': y})
df['partition'] = pd.cut(df['x'], bins=10, labels=False)
result = df.groupby('partition').mean()
memory_usage_end = memory_usage()[0]
end_time = time.time()
# 输出结果
print('运行时间:{:.4f}秒'.format(end_time - start_time))
print('所占内存:{:.2f}MB'.format(memory_usage_end - memory_usage_start))
# 绘制图表
plt.scatter(x, y, s=1, alpha=0.5)
plt.scatter(result['x'], result['y'], s=10, c='r')
plt.show()
```
希望这个回答能够帮助你解决问题!
阅读全文
相关推荐
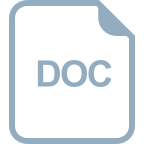
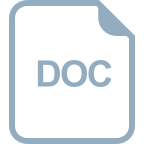





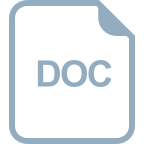
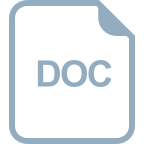
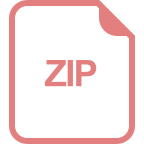
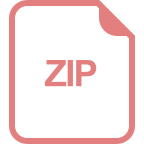
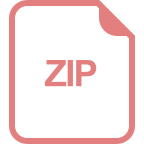
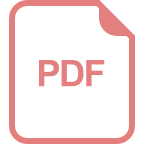