C++判断字符串中中文字符的编码格式
时间: 2023-07-14 21:12:36 浏览: 391
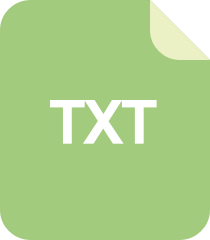
判断字符编码格式代码

在 C++ 中,可以使用以下方法来判断字符串中的中文字符的编码格式:
1. UTF-8 编码:判断一个字符是否为中文字符,可以通过检查其字节数来实现。UTF-8 中,一个中文字符占用 3 个字节,因此可以检查每个字符的字节数,如果是 3,则认为是中文字符。
```c++
bool isChineseCharacter_UTF8(const std::string& str, int index) {
if ((str[index] & 0xE0) == 0xE0) { // 判断字符的字节数
return true;
}
return false;
}
```
2. GBK 编码:GBK 编码中,一个中文字符占用 2 个字节。可以使用以下方法来判断:
```c++
bool isChineseCharacter_GBK(const std::string& str, int index) {
unsigned char c1 = str[index];
unsigned char c2 = str[index + 1];
if (c1 >= 0xA1 && c1 <= 0xF7 && c2 >= 0xA1 && c2 <= 0xFE) {
return true;
}
return false;
}
```
以上代码仅供参考,具体的实现还需要根据实际情况进行调整。
阅读全文
相关推荐


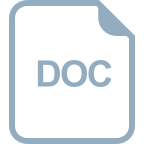



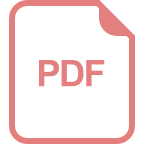
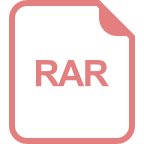
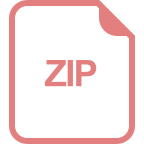
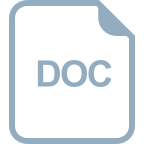
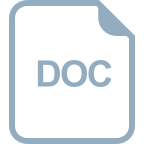
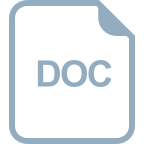
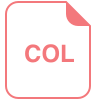


