用java写一个五子棋
时间: 2024-01-08 12:46:41 浏览: 31
好的,以下是一个简单的五子棋的 Java 实现。
```java
import java.util.Scanner;
public class Gomoku {
private static int ROWS = 15;
private static int COLS = 15;
private static char BLANK = '-';
private static char BLACK = 'X';
private static char WHITE = 'O';
private static char[][] board = new char[ROWS][COLS];
private static Scanner sc = new Scanner(System.in);
public static void main(String[] args) {
initBoard();
printBoard();
char player = BLACK;
while (true) {
System.out.println("Player " + player + "'s turn.");
int row = getValidInput("Enter row: ");
int col = getValidInput("Enter col: ");
if (board[row][col] != BLANK) {
System.out.println("This cell is occupied. Please select another cell.");
continue;
}
board[row][col] = player;
printBoard();
if (checkWin(row, col)) {
System.out.println("Player " + player + " wins!");
break;
}
if (isBoardFull()) {
System.out.println("The game is a draw!");
break;
}
player = (player == BLACK) ? WHITE : BLACK;
}
}
private static void initBoard() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
board[i][j] = BLANK;
}
}
}
private static void printBoard() {
System.out.print(" ");
for (int j = 0; j < COLS; j++) {
System.out.print(j + " ");
}
System.out.println();
for (int i = 0; i < ROWS; i++) {
System.out.print(i + " ");
for (int j = 0; j < COLS; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
private static int getValidInput(String prompt) {
int input;
while (true) {
System.out.print(prompt);
try {
input = sc.nextInt();
if (input < 0 || input >= ROWS) {
System.out.println("Invalid input. Please enter a number between 0 and " + (ROWS - 1) + ".");
continue;
}
break;
} catch (Exception e) {
System.out.println("Invalid input. Please enter a number between 0 and " + (ROWS - 1) + ".");
sc.nextLine();
}
}
return input;
}
private static boolean checkWin(int row, int col) {
char player = board[row][col];
int count;
// check horizontal
count = 1;
for (int j = col - 1; j >= 0 && board[row][j] == player; j--) {
count++;
}
for (int j = col + 1; j < COLS && board[row][j] == player; j++) {
count++;
}
if (count >= 5) {
return true;
}
// check vertical
count = 1;
for (int i = row - 1; i >= 0 && board[i][col] == player; i--) {
count++;
}
for (int i = row + 1; i < ROWS && board[i][col] == player; i++) {
count++;
}
if (count >= 5) {
return true;
}
// check diagonal (top-left to bottom-right)
count = 1;
for (int i = row - 1, j = col - 1; i >= 0 && j >= 0 && board[i][j] == player; i--, j--) {
count++;
}
for (int i = row + 1, j = col + 1; i < ROWS && j < COLS && board[i][j] == player; i++, j++) {
count++;
}
if (count >= 5) {
return true;
}
// check diagonal (bottom-left to top-right)
count = 1;
for (int i = row + 1, j = col - 1; i < ROWS && j >= 0 && board[i][j] == player; i++, j--) {
count++;
}
for (int i = row - 1, j = col + 1; i >= 0 && j < COLS && board[i][j] == player; i--, j++) {
count++;
}
if (count >= 5) {
return true;
}
return false;
}
private static boolean isBoardFull() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == BLANK) {
return false;
}
}
}
return true;
}
}
```
这个实现中,我们使用了一个二维 char 数组来表示棋盘,其中 `-` 表示空格,`X` 表示黑棋,`O` 表示白棋。我们在 `main` 方法中实现了游戏的主要逻辑:
1. 初始化棋盘并打印出来。
2. 循环进行游戏,每次轮到一个玩家时,要求玩家输入下棋的坐标。
3. 检查该位置是否已经被占据,如果被占据则提示玩家重新选择。
4. 将该位置标记为玩家的棋子,并打印出来。
5. 检查是否有玩家获胜或者棋盘已满,如果是则结束游戏。
6. 轮到另一个玩家继续游戏。
我们还实现了一些辅助方法:
- `printBoard`:打印出当前的棋盘。
- `getValidInput`:从标准输入中获取玩家输入的坐标,并进行合法性检查。
- `checkWin`:检查当前玩家是否获胜。
- `isBoardFull`:检查棋盘是否已满。
相关推荐
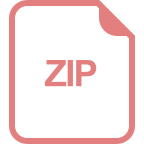
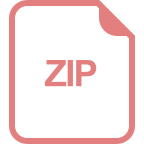














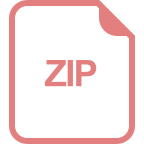