Why the following programs will cause error? #include <iostream> #include <string> using namespace std;class Box { int capacity; public: Box(int cap){ capacity = cap; } friend void show(); }; void Box::show() { Box b(10); cout<<"Value of capacity is: "<<b.capacity<<endl; } int main(){ show(); return 0; }
时间: 2024-01-14 14:02:50 浏览: 26
The program will cause an error because the `show()` function is defined outside of the `Box` class but is not declared as a friend function inside the class. To fix it, you can add a friend declaration inside the class like this:
```
class Box {
int capacity;
public:
Box(int cap){ capacity = cap; }
friend void show();
};
void show() {
Box b(10);
cout<<"Value of capacity is: "<<b.capacity<<endl;
}
int main(){
show();
return 0;
}
```
Alternatively, you can define the `show()` function inside the `Box` class like this:
```
class Box {
int capacity;
public:
Box(int cap){ capacity = cap; }
void show() {
cout<<"Value of capacity is: "<<capacity<<endl;
}
};
int main(){
Box b(10);
b.show();
return 0;
}
```
相关推荐
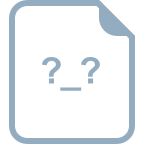














