策略模式 电商 java示例
时间: 2023-09-01 15:07:29 浏览: 148
策略模式(Strategy Pattern)是一种行为型设计模式,它允许在运行时选择算法的行为。在该模式中,算法被封装在独立的策略类中,客户端代码可以通过组合和委托来实现不同的行为。
在电商系统中,可以使用策略模式来实现不同的促销策略,如满减、折扣、赠品等。下面是一个简单的Java示例:
首先,我们定义一个促销策略接口:
```
public interface PromotionStrategy {
double applyDiscount(double price);
}
```
然后,我们实现不同的促销策略:
```
public class DiscountPromotionStrategy implements PromotionStrategy {
private double discount;
public DiscountPromotionStrategy(double discount) {
this.discount = discount;
}
@Override
public double applyDiscount(double price) {
return price * discount;
}
}
public class FullReductionPromotionStrategy implements PromotionStrategy {
private double full;
private double reduction;
public FullReductionPromotionStrategy(double full, double reduction) {
this.full = full;
this.reduction = reduction;
}
@Override
public double applyDiscount(double price) {
if (price >= full) {
return price - reduction;
}
return price;
}
}
public class GiftPromotionStrategy implements PromotionStrategy {
private String gift;
public GiftPromotionStrategy(String gift) {
this.gift = gift;
}
@Override
public double applyDiscount(double price) {
System.out.println("送您一份礼物:" + gift);
return price;
}
}
```
最后,我们在购物车类中使用策略模式来选择不同的促销策略:
```
public class ShoppingCart {
private List<Double> items = new ArrayList<>();
private PromotionStrategy promotionStrategy;
public void addItem(double price) {
items.add(price);
}
public void setPromotionStrategy(PromotionStrategy promotionStrategy) {
this.promotionStrategy = promotionStrategy;
}
public double calculateTotal() {
double total = items.stream().mapToDouble(Double::doubleValue).sum();
if (promotionStrategy != null) {
total = promotionStrategy.applyDiscount(total);
}
return total;
}
}
```
使用示例:
```
ShoppingCart cart = new ShoppingCart();
cart.addItem(100);
cart.addItem(200);
cart.addItem(300);
// 使用折扣策略
cart.setPromotionStrategy(new DiscountPromotionStrategy(0.8));
double total1 = cart.calculateTotal();
System.out.println("折扣后总价:" + total1); // 折扣后总价:480.0
// 使用满减策略
cart.setPromotionStrategy(new FullReductionPromotionStrategy(500, 100));
double total2 = cart.calculateTotal();
System.out.println("满减后总价:" + total2); // 满减后总价:500.0
// 使用赠品策略
cart.setPromotionStrategy(new GiftPromotionStrategy("小熊玩具"));
double total3 = cart.calculateTotal();
System.out.println("赠品后总价:" + total3); // 赠品后总价:600.0
```
这样,我们就可以根据需求灵活地选择不同的促销策略,而不需要修改购物车类的代码。
阅读全文
相关推荐




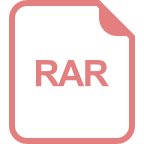













