用python对twitter数据情感分析
时间: 2024-02-21 11:02:05 浏览: 28
在Python中进行Twitter数据情感分析,可以使用一些第三方库和工具来实现。以下是一个基本的步骤:
1. 获取Twitter数据:可以使用Twitter API来获取Twitter数据,或者使用一些第三方工具,如Tweepy等。
2. 数据预处理:对获取的Twitter数据进行预处理,包括去除停用词、标点符号和URL链接等,以及进行分词和词性标注等。
3. 情感分析:使用情感分析算法对预处理后的Twitter文本进行情感分类和打分,通常情感分析算法包括基于规则的方法、情感词典方法以及机器学习方法等。
4. 结果可视化:将情感分析结果可视化展示,如柱状图、饼状图等。
以下是一个使用Python进行Twitter数据情感分析的示例代码:
```python
import tweepy
from textblob import TextBlob
import matplotlib.pyplot as plt
# Twitter API credentials
consumer_key = "your_consumer_key"
consumer_secret = "your_consumer_secret"
access_key = "your_access_key"
access_secret = "your_access_secret"
# Authenticate to Twitter
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_key, access_secret)
# Create API object
api = tweepy.API(auth)
# Search for tweets containing a certain keyword
public_tweets = api.search('Python')
# Initialize variables to store sentiment scores
positive = 0
negative = 0
neutral = 0
# Loop through each tweet and perform sentiment analysis
for tweet in public_tweets:
analysis = TextBlob(tweet.text)
if analysis.sentiment.polarity > 0:
positive += 1
elif analysis.sentiment.polarity < 0:
negative += 1
else:
neutral += 1
# Visualize the sentiment analysis results
labels = ['Positive', 'Negative', 'Neutral']
sizes = [positive, negative, neutral]
colors = ['yellowgreen', 'lightcoral', 'gold']
explode = (0.1, 0, 0)
plt.pie(sizes, explode=explode, labels=labels, colors=colors,
autopct='%1.1f%%', shadow=True, startangle=140)
plt.axis('equal')
plt.show()
```
上述代码使用Tweepy库获取包含关键词“Python”的Twitter数据,使用TextBlob库进行情感分析,然后使用Matplotlib库将情感分析结果可视化展示。
相关推荐
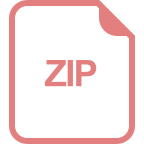














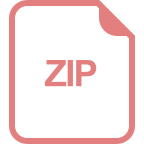