c++灰度图像线性拉伸不用opencv
时间: 2023-07-19 16:06:01 浏览: 56
线性拉伸是一种简单的灰度图像增强方法,可以通过对图像像素值进行调整来增强图像的对比度和亮度。以下是一个使用C++实现灰度图像线性拉伸的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
struct Image {
int width; // 图像宽度
int height; // 图像高度
vector<unsigned char> data; // 像素值
};
// 读取PGM格式图像
void read_pgm(const char* filename, Image& img) {
ifstream file(filename, ios::binary); // 以二进制模式打开文件
if (!file) {
cerr << "Error: cannot open file " << filename << endl;
exit(1);
}
// 读取文件头
string magic_number;
file >> magic_number;
if (magic_number != "P5") {
cerr << "Error: not a PGM file" << endl;
exit(1);
}
file >> img.width >> img.height;
int max_value;
file >> max_value;
if (max_value != 255) {
cerr << "Error: not a 8-bit PGM file" << endl;
exit(1);
}
file.get(); // 跳过换行符
// 读取像素值
img.data.resize(img.width * img.height);
file.read(reinterpret_cast<char*>(&img.data[0]), img.data.size());
}
// 写入PGM格式图像
void write_pgm(const char* filename, const Image& img) {
ofstream file(filename, ios::binary); // 以二进制模式打开文件
if (!file) {
cerr << "Error: cannot open file " << filename << endl;
exit(1);
}
// 写入文件头
file << "P5\n" << img.width << ' ' << img.height << "\n255\n";
// 写入像素值
file.write(reinterpret_cast<const char*>(&img.data[0]), img.data.size());
}
// 线性拉伸
void linear_stretch(Image& img) {
// 计算像素值的最小值和最大值
unsigned char min_value = *min_element(img.data.begin(), img.data.end());
unsigned char max_value = *max_element(img.data.begin(), img.data.end());
// 线性拉伸
for (unsigned char& pixel : img.data) {
pixel = (pixel - min_value) * 255.0 / (max_value - min_value);
}
}
int main() {
Image img;
read_pgm("test.pgm", img);
linear_stretch(img);
write_pgm("result.pgm", img);
return 0;
}
```
该代码使用STL库中的vector存储像素值,通过读取PGM格式图像文件来获取图像的宽度、高度和像素值,然后计算像素值的最小值和最大值,并进行线性拉伸处理,最后将处理后的像素值写入PGM格式图像文件。要使用该代码,只需将读取和写入文件的文件名替换为您要处理的图像文件名即可。
相关推荐
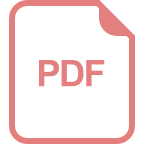










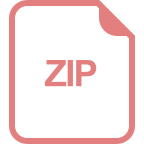