python 用re查找字符串
时间: 2023-12-31 16:06:43 浏览: 81
使用Python中的re模块可以查找字符串中的特定模式。以下是一个简单的示例,使用re模块在字符串中查找所有以字母"p"开头,后跟任意数量的字母和数字的单词:
```python
import re
# 定义一个字符串,包含一些单词
text = "peter piper picked a peck of pickled peppers"
# 定义要查找的模式,使用正则表达式
pattern = r"p\w+"
# 使用re模块中的findall函数查找所有匹配的字符串
result = re.findall(pattern, text)
# 输出结果
print(result) # ['peter', 'piper', 'picked', 'peck', 'pickled', 'peppers']
```
在上面的示例中,使用正则表达式模式`r"p\w+"`匹配所有以字母"p"开头,后跟任意数量的字母和数字的单词。然后使用re模块中的findall函数查找所有匹配的字符串,并将结果存储在变量result中。最后,将结果输出到控制台。
相关问题
python3 查找字符串
在Python 3中,可以使用多种方法来查找字符串。以下是几种常用的方法:
1. 使用in关键字:可以使用in关键字来检查一个字符串是否包含另一个字符串。例如:
```python
string = "Hello, World!"
if "Hello" in string:
print("Found")
else:
print("Not found")
```
2. 使用find()方法:find()方法可以用来查找一个子字符串在另一个字符串中的位置。如果找到了,则返回子字符串的起始索引;如果没有找到,则返回-1。例如:
```python
string = "Hello, World!"
index = string.find("World")
if index != -1:
print("Found at index", index)
else:
print("Not found")
```
3. 使用index()方法:index()方法与find()方法类似,但是如果子字符串不存在于原字符串中,会抛出ValueError异常。例如:
```python
string = "Hello, World!"
try:
index = string.index("World")
print("Found at index", index)
except ValueError:
print("Not found")
```
4. 使用正则表达式:如果需要更复杂的模式匹配,可以使用Python的re模块来进行正则表达式匹配。例如:
```python
import re
string = "Hello, World!"
pattern = r"World"
match = re.search(pattern, string)
if match:
print("Found at index", match.start())
else:
print("Not found")
```
这些是Python 3中常用的字符串查找方法。希望对你有帮助!
python正则匹配查找字符串的代码
可以使用 re 模块来进行正则匹配,比如通过 re.search() 函数来查找字符串中是否包含特定的模式。例如:
import re
pattern = r'hello'
string = 'hello world'
match = re.search(pattern, string)
if match:
print("Found a match!")
else:
print("No match found.")
阅读全文
相关推荐
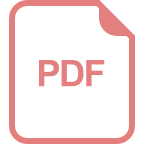
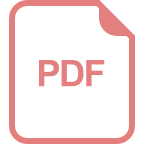
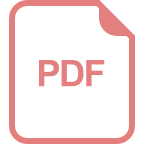













