写一个程序,定义抽象基类Shape,由它派生出3个派生类:Circle(圆形)、Square(正方形)、Rectangle(矩形)。利用指针、虚函数printArea()、printLength()分别计算以上三者的面积和周长,三个图形的数据在定义对象时给定。另外实现一个通用的函数输出面积和周长,而不需要考虑是何种图形。
时间: 2024-03-23 21:38:48 浏览: 76
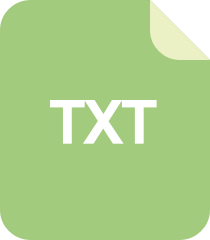
声明一个图形基类并在它的基础上派生出其他类并编写程序实现各类的定义及实现
下面是一个简单的实现:
```C++
#include <iostream>
#include <string>
using namespace std;
// 抽象基类 Shape,定义了两个纯虚函数
class Shape {
public:
virtual float getArea() const = 0; // 获取面积
virtual float getPerimeter() const = 0; // 获取周长
};
// Circle 类,继承自 Shape
class Circle : public Shape {
public:
Circle(float r) : radius(r) {}
// 实现 Shape 的纯虚函数
float getArea() const override {
return 3.1415 * radius * radius;
}
float getPerimeter() const override {
return 2 * 3.1415 * radius;
}
private:
float radius; // 圆的半径
};
// Square 类,继承自 Shape
class Square : public Shape {
public:
Square(float s) : side(s) {}
// 实现 Shape 的纯虚函数
float getArea() const override {
return side * side;
}
float getPerimeter() const override {
return 4 * side;
}
private:
float side; // 正方形的边长
};
// Rectangle 类,继承自 Shape
class Rectangle : public Shape {
public:
Rectangle(float w, float h) : width(w), height(h) {}
// 实现 Shape 的纯虚函数
float getArea() const override {
return width * height;
}
float getPerimeter() const override {
return 2 * (width + height);
}
private:
float width; // 矩形的宽
float height; // 矩形的高
};
// 输出面积和周长的通用函数
void printInfo(const Shape& shape) {
cout << "Area: " << shape.getArea() << endl;
cout << "Perimeter: " << shape.getPerimeter() << endl;
}
int main() {
Circle c(5);
Square s(6);
Rectangle r(6, 8);
cout << "Circle:" << endl;
printInfo(c);
cout << "Square:" << endl;
printInfo(s);
cout << "Rectangle:" << endl;
printInfo(r);
return 0;
}
```
其中,`Shape` 是一个抽象基类,定义了两个纯虚函数 `getArea` 和 `getPerimeter`,分别用于获取图形的面积和周长。`Circle`、`Square`、`Rectangle` 三个派生类分别继承自 `Shape`,并实现了 `getArea` 和 `getPerimeter` 函数。`printInfo` 函数是一个通用的输出面积和周长的函数,可以接受任何继承自 `Shape` 的对象作为参数。
在 `main` 函数中,分别创建一个 `Circle`、一个 `Square` 和一个 `Rectangle` 对象,并传入 `printInfo` 函数中,输出它们的面积和周长。
阅读全文
相关推荐
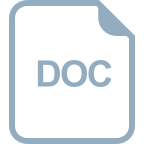
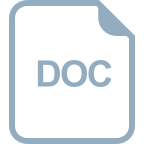










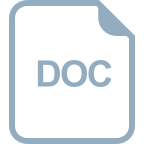
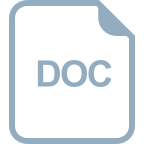
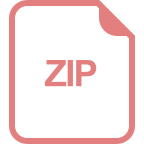
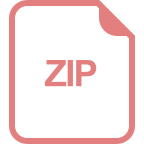