ifstream readFile
时间: 2023-07-27 10:11:04 浏览: 101
这是一个 C++ 语言中的代码,用来创建一个输入文件流对象。它可以打开一个文本文件,并从中读取数据。
示例代码:
```c++
#include <fstream>
using namespace std;
int main() {
ifstream readFile("example.txt"); // 创建输入文件流对象
if (readFile.is_open()) { // 检查文件是否成功打开
string line;
while (getline(readFile, line)) { // 逐行读取文件内容
cout << line << endl;
}
readFile.close(); // 关闭文件流
}
return 0;
}
```
以上代码会打开一个名为 "example.txt" 的文本文件,并逐行读取其中的内容。如果文件成功打开,则会输出文件的每一行。最后,文件流对象会被关闭。
相关问题
ifstream readfile
ifstream readfile是一个C++中的输入流对象,用于从文件中读取数据。通过该对象,可以打开一个文件,并从文件中读取内容。
要使用ifstream对象readfile,首先需要包含头文件<fstream>。然后,可以使用以下语法打开文件并读取内容:
```cpp
#include <fstream>
using namespace std;
int main() {
ifstream readfile("filename.txt");
if (readfile.is_open()) {
// 文件打开成功
// 在这里进行文件读取操作
} else {
// 文件打开失败
cout << "无法打开文件" << endl;
}
readfile.close(); // 关闭文件
return 0;
}
```
上述代码中,我们使用ifstream对象readfile打开了一个名为"filename.txt"的文件。然后,我们使用is_open()函数验证文件是否成功打开。如果成功打开,可以在if语句块内进行文件读取操作。否则,输出错误消息。
ifstream readFile() 地址
`ifstream` 是 C++ 标准库中的一个输入流类,用于读取文件。`readFile()` 不仅需要返回一个 `ifstream` 对象,还需要指定要打开的文件的地址。因此,可以这样实现 `readFile()` 函数:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
ifstream readFile(const string& filePath) {
ifstream file(filePath);
if (!file.is_open()) {
cerr << "Error opening file " << filePath << endl;
exit(1);
}
return file;
}
int main() {
const string filePath = "example.txt";
ifstream file = readFile(filePath);
// 从文件中读取数据
file.close();
return 0;
}
```
在上面的代码中,`readFile()` 函数使用 `ifstream` 类创建一个文件输入流对象,并尝试打开指定路径下的文件。如果文件打开失败,则输出错误信息并退出程序。如果文件打开成功,则返回该文件输入流对象。在 `main()` 函数中,可以通过调用 `readFile()` 函数来获取文件输入流对象,并使用该对象进行文件操作。最后,记得关闭文件输入流对象。
阅读全文
相关推荐




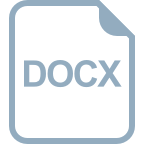
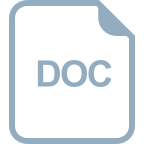







