.定义一个名为employee的类,它继承person类,其中定义salary(表示工资)和department(表示部门)两个成员变量和封装这两个变量的方法。编写主程序检查新建类中的所有方法。
时间: 2023-05-31 21:19:22 浏览: 131
### 回答1:
这是一个关于员工的类,它继承自person类,其中定义了salary(表示工资)和department(表示部门)两个成员变量,还有两个成员变量的setter和getter方法。编写主程序时需要检查新建类中的所有方法。
### 回答2:
Employee类是继承自Person类的一个子类,它有两个成员变量:salary和department,用来表示员工的工资和部门。为了保证类的封装性,应该对这两个成员变量采用私有化处理,同时提供公有的setter和getter方法供外部使用来访问和修改这两个数据成员。这样做的好处是可以根据需要对数据进行有效的控制,而不会影响其他部分的代码。
下面是Employee类的代码实现:
```java
public class Employee extends Person {
private double salary;
private String department;
public Employee(String name, int age, String gender, double salary, String department) {
super(name, age, gender);
this.salary = salary;
this.department = department;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
public void display() {
super.display();
System.out.println("Salary: " + salary + " Department: " + department);
}
}
```
在Employee类的构造函数中,用super关键字来调用父类Person的构造函数,初始化继承自Person类的成员变量name、age和gender。同时,Employee类新增了salary和department两个成员变量。
由于Person类已经实现了display()方法,因此在Employee类中可以直接使用super.display()来调用Person类的display()方法,从而打印出继承自Person类的成员变量。Employee类新增的成员变量salary和department也被一并打印出来。
主程序中可以如下来检查Employee类:
```java
public class Main {
public static void main(String[] args) {
Employee e = new Employee("Tom", 25, "male", 5000, "sales department");
e.display();
e.setSalary(6000);
e.setDepartment("marketing department");
e.display();
}
}
```
在主程序中,首先创建一个Employee对象并初始化其成员变量。然后使用对象的display()方法打印出员工的信息。之后分别使用setter方法修改对象的salary和department成员变量,并通过对象的display()方法再次打印出修改后的员工信息,用来验证setter方法是否起作用。
### 回答3:
定义一个名为employee的类,它继承person类,可以理解为employee类拥有person类的所有属性和方法。类中定义了salary(表示工资)和department(表示部门)两个成员变量。因为salary和department是私有成员变量,所以我们需要在类中封装这两个变量的方法。
一个方法是set方法,用于给salary和department赋值。set方法的定义如下:
```python
def set_employee_info(self, salary, department):
self.salary = salary
self.department = department
```
另一个方法是get方法,用于获取salary和department的值。get方法的定义如下:
```python
def get_employee_info(self):
return {'salary': self.salary, 'department': self.department}
```
现在,我们可以编写一个主程序来检查新建类中的所有方法。首先,我们需要导入Person类,然后创建一个Employee类的对象,该对象继承了Person类的属性和方法。
```python
from person import Person
class Employee(Person):
def __init__(self, name, age):
super().__init__(name, age)
self.salary = 0
self.department = ''
def set_employee_info(self, salary, department):
self.salary = salary
self.department = department
def get_employee_info(self):
return {'salary': self.salary, 'department': self.department}
```
现在,我们可以使用Employee类创建对象,并调用其方法。例如:
```python
e = Employee('Tom', 25)
e.set_employee_info(5000, 'Sales')
print(e.get_employee_info())
```
以上代码的输出应该是:
```
{'salary': 5000, 'department': 'Sales'}
```
这说明我们编写的Employee类中的方法都有效。我们可以通过这样的方式来验证类中所有方法是否都有效。
相关推荐
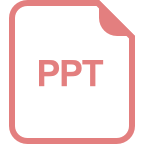
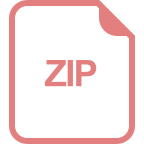
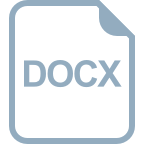














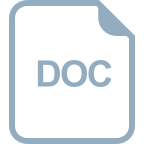