使用类计算三角形面积,在类函数中判断如不能构成三角形抛出异常结束,否则可继续循环输入三角形三边计算,直到输入N/n结束,输入在主函数中进行
时间: 2024-05-12 18:15:23 浏览: 14
以下是使用类计算三角形面积的示例代码:
```c++
#include <iostream>
#include <cmath>
#include <string>
#include <stdexcept>
using namespace std;
class Triangle {
private:
double a, b, c;
public:
Triangle(double _a, double _b, double _c) : a(_a), b(_b), c(_c) {}
double getArea() {
if (a + b <= c || a + c <= b || b + c <= a) {
throw invalid_argument("Invalid triangle sides!");
}
double p = (a + b + c) / 2;
return sqrt(p * (p - a) * (p - b) * (p - c));
}
};
int main() {
string input;
while (true) {
cout << "Enter triangle sides (separated by space) or N/n to exit: ";
getline(cin, input);
if (input == "N" || input == "n") {
break;
}
double a, b, c;
if (sscanf(input.c_str(), "%lf %lf %lf", &a, &b, &c) != 3) {
cout << "Invalid input format!" << endl;
continue;
}
try {
Triangle triangle(a, b, c);
cout << "Triangle area: " << triangle.getArea() << endl;
} catch (const invalid_argument& e) {
cout << "Error: " << e.what() << endl;
}
}
return 0;
}
```
在上述代码中,`Triangle` 类表示一个三角形,其中 `a`、`b`、`c` 分别表示三角形的三条边长。`getArea` 函数用于计算三角形的面积,如果三条边长不能构成一个三角形,则抛出 `invalid_argument` 异常。
在主函数中,使用 `getline` 函数读取用户输入的字符串,然后使用 `sscanf` 函数解析出三条边长。如果解析失败,提示用户输入格式错误并继续循环。如果解析成功,创建一个 `Triangle` 对象并调用 `getArea` 函数计算面积。如果抛出了异常,则捕获异常并输出错误信息。如果用户输入了 N/n,则退出循环。
相关推荐
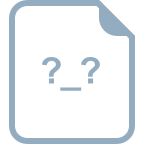
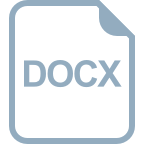
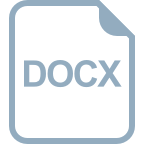












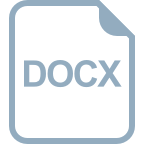
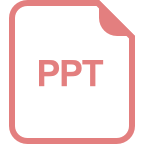