LL(1)分析、LR分析和算符优先分析的区别
时间: 2023-10-10 10:08:45 浏览: 221
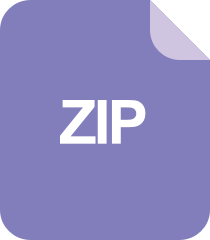
编译原理课程设计-LL(1)预测分析法 算符优先分析 LR分析

LL(1)分析、LR分析和算符优先分析都是语法分析算法,它们的主要区别在于:
1. LL(1)分析是自顶向下的语法分析算法,LR分析是自底向上的语法分析算法,而算符优先分析是一种特殊的自底向上的语法分析算法。
2. LL(1)分析和LR分析都是基于产生式的语法分析算法,而算符优先分析则是基于算符优先级和关联性的语法分析算法。
3. LL(1)分析和LR分析都可以处理相对复杂的文法,而算符优先分析通常只适用于处理表达式文法等简单的文法。
4. LL(1)分析和LR分析都需要构造分析表,而算符优先分析则需要构造算符优先关系表。
5. LL(1)分析和LR分析都可以采用自动机进行实现,而算符优先分析则可以采用递归下降或移进-规约方式进行实现。
总的来说,LL(1)分析、LR分析和算符优先分析都有各自的适用范围和优缺点,要根据具体的文法和应用场景选择合适的语法分析算法。
阅读全文
相关推荐
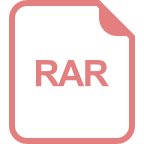
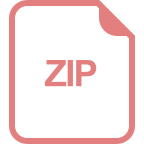
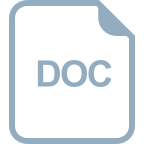




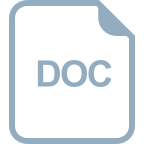
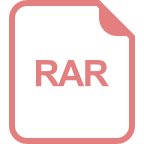
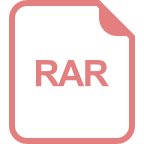
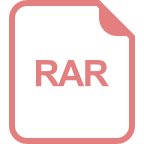
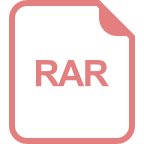
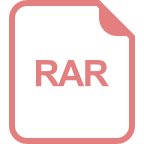
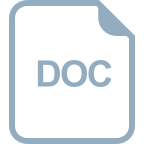
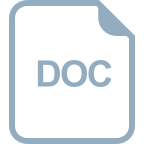


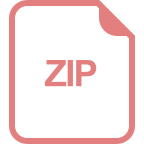
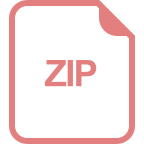