this.$confirm 确定时的返回值
时间: 2023-07-06 10:16:48 浏览: 84
`this.$confirm` 返回的是一个Promise对象,你可以使用`.then()`方法来获取用户在弹出框中点击的按钮类型。`.then()`方法接收一个回调函数,该回调函数的第一个参数为用户点击的按钮类型,包括`confirm`和`cancel`。
例如,以下是一个示例代码:
```
this.$confirm('确定要删除吗?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
// 用户点击了确定按钮
console.log('用户点击了确定按钮');
}).catch(() => {
// 用户点击了取消按钮
console.log('用户点击了取消按钮');
});
```
在这个示例中,如果用户点击了确定按钮,控制台将输出`用户点击了确定按钮`;如果用户点击了取消按钮,控制台将输出`用户点击了取消按钮`。
相关问题
this.$root.$confirm
this.$root.$confirm是一个Vue.js中的方法,用于显示一个确认对话框。它的具体实现可以根据不同的项目和组件库而有所不同。根据提供的引用内容,我们可以看到在引用[1]和引用[2]中都使用了this.$confirm方法来显示确认对话框。这个方法接受三个参数:确认消息、对话框标题和选项对象。选项对象中可以设置确认按钮的文本、取消按钮的文本和对话框的类型。当用户点击确认按钮时,会执行then回调函数,而当用户点击取消按钮时,会执行catch回调函数。在这些回调函数中,可以执行相应的操作,比如显示成功或取消的提示消息。根据提供的引用内容,我们可以看到在确认对话框的then回调函数中显示了成功的提示消息,而在catch回调函数中显示了取消的提示消息。所以,this.$root.$confirm方法可以用于显示一个确认对话框,并根据用户的选择执行相应的操作。
如何销毁 this.$confirm
this.$confirm是Element UI库中的一个方法,用于显示一个确认对话框。销毁this.$confirm实际上是关闭这个对话框。
要销毁this.$confirm,可以使用以下方法之一:
1. 调用返回的Promise对象的cancel方法:this.$confirm方法返回一个Promise对象,可以通过调用该对象的cancel方法来关闭对话框。例如:
```
const confirmInstance = this.$confirm('确定要删除吗?');
confirmInstance.cancel();
```
2. 调用返回的Promise对象的finally方法:Promise对象的finally方法会在Promise对象状态改变后执行,无论是resolve还是reject。可以在finally方法中关闭对话框。例如:
```
const confirmInstance = this.$confirm('确定要删除吗?');
confirmInstance.finally(() => {
// 关闭对话框的逻辑
});
```
3. 使用Vue的$nextTick方法:Vue的$nextTick方法可以在DOM更新后执行回调函数。可以在回调函数中关闭对话框。例如:
```
const confirmInstance = this.$confirm('确定要删除吗?');
this.$nextTick(() => {
// 关闭对话框的逻辑
});
```
相关推荐
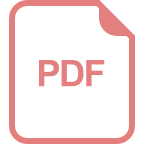
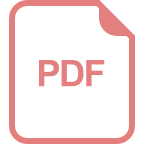












