编写并调试一个模拟的进程调度程序,采用 “短 进程优先”调度算法对多个进程进行调度。 计算平均周转时间和平均带权周转时间。
时间: 2023-07-31 16:14:10 浏览: 42
好的,我可以给你提供一个简单的示例代码,用于模拟短进程优先调度算法。
首先,我们需要定义一个进程的结构体,包括进程ID、到达时间、运行时间、完成时间、等待时间、周转时间和带权周转时间等信息。
```c
typedef struct {
int pid; // 进程ID
int arrive_time; // 到达时间
int run_time; // 运行时间
int finish_time; // 完成时间
int wait_time; // 等待时间
float turnaround_time; // 周转时间
float weighted_turnaround_time; // 带权周转时间
} Process;
```
接下来,我们需要实现一个比较函数,用于按照进程的运行时间进行升序排序,以便后续进行调度。
```c
int compare(const void *a, const void *b) {
Process *p1 = (Process *)a;
Process *p2 = (Process *)b;
return p1->run_time - p2->run_time;
}
```
然后,我们可以编写主函数,模拟短进程优先调度算法。具体步骤如下:
1. 读入进程数和每个进程的信息。
2. 按照到达时间对进程进行排序。
3. 定义一个队列,用于存储已到达但尚未运行的进程。
4. 定义一个当前时间变量,初始化为第一个进程的到达时间。
5. 循环进行调度,直到所有进程都完成。
6. 在每个时间点,将已到达但尚未运行的进程加入队列中。
7. 从队列中选出运行时间最短的进程进行运行。
8. 更新当前时间和进程信息,并将已完成的进程从队列中移除。
9. 计算每个进程的周转时间和带权周转时间,并计算平均值。
下面是完整的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int pid; // 进程ID
int arrive_time; // 到达时间
int run_time; // 运行时间
int finish_time; // 完成时间
int wait_time; // 等待时间
float turnaround_time; // 周转时间
float weighted_turnaround_time; // 带权周转时间
} Process;
int compare(const void *a, const void *b) {
Process *p1 = (Process *)a;
Process *p2 = (Process *)b;
return p1->run_time - p2->run_time;
}
int main() {
int n;
printf("请输入进程数:");
scanf("%d", &n);
Process *processes = (Process *)malloc(n * sizeof(Process));
for (int i = 0; i < n; i++) {
printf("请输入第%d个进程的到达时间和运行时间:", i + 1);
scanf("%d%d", &processes[i].arrive_time, &processes[i].run_time);
processes[i].pid = i + 1;
}
qsort(processes, n, sizeof(Process), compare);
int current_time = processes[0].arrive_time;
int completed = 0;
int idx = 0;
Process *queue = (Process *)malloc(n * sizeof(Process));
while (completed < n) {
while (idx < n && processes[idx].arrive_time <= current_time) {
queue[idx] = processes[idx];
idx++;
}
if (idx == 0) {
current_time = processes[idx].arrive_time;
continue;
}
int min_idx = 0;
for (int i = 1; i < idx; i++) {
if (queue[i].run_time < queue[min_idx].run_time) {
min_idx = i;
}
}
Process p = queue[min_idx];
p.wait_time = current_time - p.arrive_time;
p.finish_time = current_time + p.run_time;
p.turnaround_time = (float)(p.finish_time - p.arrive_time);
p.weighted_turnaround_time = p.turnaround_time / p.run_time;
current_time = p.finish_time;
completed++;
for (int i = min_idx; i < idx - 1; i++) {
queue[i] = queue[i + 1];
}
idx--;
processes[p.pid - 1] = p;
}
printf("进程ID\t到达时间\t运行时间\t完成时间\t等待时间\t周转时间\t带权周转时间\n");
float avg_turnaround_time = 0.0;
float avg_weighted_turnaround_time = 0.0;
for (int i = 0; i < n; i++) {
Process p = processes[i];
printf("%d\t%d\t\t%d\t\t%d\t\t%d\t\t%.2f\t\t%.2f\n", p.pid, p.arrive_time,
p.run_time, p.finish_time, p.wait_time, p.turnaround_time, p.weighted_turnaround_time);
avg_turnaround_time += p.turnaround_time;
avg_weighted_turnaround_time += p.weighted_turnaround_time;
}
avg_turnaround_time /= n;
avg_weighted_turnaround_time /= n;
printf("平均周转时间:%.2f\n", avg_turnaround_time);
printf("平均带权周转时间:%.2f\n", avg_weighted_turnaround_time);
free(processes);
free(queue);
return 0;
}
```
运行程序后,输入进程数和每个进程的到达时间和运行时间,即可计算出平均周转时间和平均带权周转时间。
相关推荐
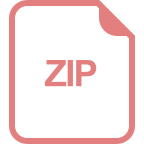
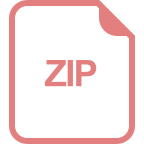
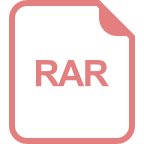














