Canvas { id: canvas // ... Rectangle { id: rect x: 100 y: 100 width: 50 height: 50 color: "red" // 当选中该图形时,添加一个SelectionBox元素 property bool selected: false MouseArea { anchors.fill: parent onClicked: { rect.selected = true; } } // 监听属性变化,添加或移除SelectionBox元素 onSelectedChanged: { if (selected) { var selectionBox = canvas.createSelectionBox(rect); selectionBox.rotation = rect.rotation; selectionBox.scaleX = rect.scaleX; selectionBox.scaleY = rect.scaleY; } else { canvas.removeSelectionBox(rect); } } } function createSelectionBox(item) { // ... } function removeSelectionBox(item) { // ... } }希望你完善这段代码
时间: 2024-02-10 07:14:48 浏览: 25
好的,我来帮你完善这段代码。这段代码实现了一个Canvas元素,其中包含一个红色的矩形,当该矩形被选中时,会添加一个SelectionBox元素。
首先,我们需要定义SelectionBox元素的样式和属性。在Canvas元素中添加如下代码:
```
Rectangle {
id: selectionBox
color: "transparent"
border.color: "blue"
border.width: 2
visible: false
rotation: 0
scaleX: 1
scaleY: 1
}
```
这定义了一个透明的矩形,并添加了蓝色的边框,用于表示选中状态。visible属性用于控制是否显示该元素,rotation、scaleX和scaleY属性用于同步选中的矩形的旋转角度和缩放比例。
接下来,我们需要完善Canvas元素中的createSelectionBox和removeSelectionBox方法。这两个方法分别用于创建和移除选中状态下的SelectionBox元素。
```
function createSelectionBox(item) {
if (item.parent === canvas) {
selectionBox.x = item.x - 5;
selectionBox.y = item.y - 5;
selectionBox.width = item.width + 10;
selectionBox.height = item.height + 10;
selectionBox.visible = true;
canvas.setChildIndex(selectionBox, canvas.children.length - 1);
return selectionBox;
}
}
function removeSelectionBox(item) {
if (selectionBox.parent === canvas) {
selectionBox.visible = false;
}
}
```
createSelectionBox方法会将SelectionBox元素添加到Canvas元素中,并根据选中的矩形的位置和大小设置SelectionBox的位置和大小。这里使用了Canvas元素的setChildIndex方法,将SelectionBox元素移动到Canvas元素的最上层,以确保它始终在选中的矩形的上方。
removeSelectionBox方法简单地将SelectionBox元素的visible属性设置为false,以隐藏它。
最后,我们需要修改选中矩形的onSelectedChanged方法,以调用createSelectionBox和removeSelectionBox方法。
```
onSelectedChanged: {
if (selected) {
var selectionBox = canvas.createSelectionBox(rect);
selectionBox.rotation = rect.rotation;
selectionBox.scaleX = rect.scaleX;
selectionBox.scaleY = rect.scaleY;
} else {
canvas.removeSelectionBox(rect);
}
}
```
这里直接调用createSelectionBox方法,并将返回的SelectionBox元素赋值给一个变量。然后根据选中的矩形的旋转角度和缩放比例,同步设置SelectionBox元素的旋转角度和缩放比例。如果选中状态变为false,则调用removeSelectionBox方法。
完整代码如下:
```
Canvas {
id: canvas
// ...
Rectangle {
id: rect
x: 100
y: 100
width: 50
height: 50
color: "red"
property bool selected: false
MouseArea {
anchors.fill: parent
onClicked: {
rect.selected = true;
}
}
onSelectedChanged: {
if (selected) {
var selectionBox = canvas.createSelectionBox(rect);
selectionBox.rotation = rect.rotation;
selectionBox.scaleX = rect.scaleX;
selectionBox.scaleY = rect.scaleY;
} else {
canvas.removeSelectionBox(rect);
}
}
}
Rectangle {
id: selectionBox
color: "transparent"
border.color: "blue"
border.width: 2
visible: false
rotation: 0
scaleX: 1
scaleY: 1
}
function createSelectionBox(item) {
if (item.parent === canvas) {
selectionBox.x = item.x - 5;
selectionBox.y = item.y - 5;
selectionBox.width = item.width + 10;
selectionBox.height = item.height + 10;
selectionBox.visible = true;
canvas.setChildIndex(selectionBox, canvas.children.length - 1);
return selectionBox;
}
}
function removeSelectionBox(item) {
if (selectionBox.parent === canvas) {
selectionBox.visible = false;
}
}
}
```
相关推荐
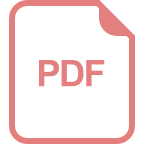
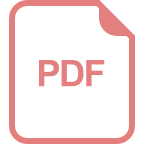
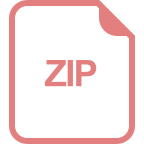














