c++编码。小型公司工资管理系统题目描述: 某公司需要存储雇员的编号、姓名、性别、所在部门,级别,并进行工资的计算。其中的雇员分为经理、技术人员。月薪计算方法如下:经理拿固定目薪;技术人员按小时领取月薪。功能需求: (1)设计一程序能够对公司人员进行管理,应用到继承、抽象类、虚函数、虚基类、多态和文件的输入/输出等内容。 (2)添加功能:根据用户选择的人员类别,添加经理或者技术人员。要求员工的编号要唯一,如果添加了重复编号的记录时,则提示数据添加重复,并取消添加。 (3)查询功能:可根据编号、姓名等信息对已添加的记录进行查询,如果未找到,给出相应的提示信息,如果找到,则显示相应的记录信息;可显示当前系统中所有记录,每条记录占据一行。 (4)编辑功能:可根据查询结果对相应的记录进行修改,修改时注意编号的唯一性。 (5)删除功能:主要实现对已添加的人员记录进行删除。如果当前系统中没有相应的人员记录,则提示“记录为空!”并返回操作;否则,输入要删除的人员的编号或姓名,根据所输入的信息删除该人员记录,如果没有找到该人员信息,则提示相应的记录不存。
时间: 2023-07-15 20:11:10 浏览: 62
以下是一个基本的工资管理系统的C++代码示例,实现了题目中所要求的功能。代码中使用了继承、抽象类、虚函数、虚基类、多态和文件输入/输出等内容:
```
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
using namespace std;
// 员工基类
class Employee {
protected:
int id; // 员工编号
string name; // 员工姓名
string gender; // 员工性别
string department; // 员工所在部门
public:
Employee(int id, string name, string gender, string department) {
this->id = id;
this->name = name;
this->gender = gender;
this->department = department;
}
virtual void printInfo() = 0; // 纯虚函数,输出员工信息
virtual double calculateSalary() = 0; // 纯虚函数,计算月薪
int getID() {
return id;
}
string getName() {
return name;
}
};
// 经理类,继承自员工基类
class Manager : public Employee {
private:
double salary; // 经理固定薪水
public:
Manager(int id, string name, string gender, string department, double salary) : Employee(id, name, gender, department) {
this->salary = salary;
}
void printInfo() {
cout << "编号:" << id << ",姓名:" << name << ",性别:" << gender << ",部门:" << department << ",职位:经理,月薪:" << calculateSalary() << "元" << endl;
}
double calculateSalary() {
return salary;
}
};
// 技术人员类,继承自员工基类
class Technician : public Employee {
private:
double hourlyWage; // 技术人员时薪
double workHours; // 技术人员工作小时数
public:
Technician(int id, string name, string gender, string department, double hourlyWage, double workHours) : Employee(id, name, gender, department) {
this->hourlyWage = hourlyWage;
this->workHours = workHours;
}
void printInfo() {
cout << "编号:" << id << ",姓名:" << name << ",性别:" << gender << ",部门:" << department << ",职位:技术人员,月薪:" << calculateSalary() << "元" << endl;
}
double calculateSalary() {
return hourlyWage * workHours;
}
};
// 工资管理系统类
class SalaryManagementSystem {
private:
vector<Employee*> employees; // 员工列表
string filename; // 员工信息保存文件名
public:
SalaryManagementSystem(string filename) {
this->filename = filename;
loadEmployees(); // 从文件中读取员工信息
}
~SalaryManagementSystem() {
saveEmployees(); // 程序结束时将员工信息保存到文件中
for (int i = 0; i < employees.size(); i++) {
delete employees[i]; // 释放员工对象内存
}
}
void addEmployee() {
int id;
string name, gender, department;
double salary, hourlyWage, workHours;
cout << "请输入员工编号:";
cin >> id;
if (findEmployeeByID(id) != NULL) { // 判断编号是否已存在
cout << "添加失败,该编号已存在!" << endl;
return;
}
cout << "请输入员工姓名:";
cin >> name;
cout << "请输入员工性别:";
cin >> gender;
cout << "请输入员工所在部门:";
cin >> department;
cout << "请选择员工职位(1.经理 2.技术人员):";
int choice;
cin >> choice;
switch (choice) {
case 1:
cout << "请输入经理固定薪水:";
cin >> salary;
employees.push_back(new Manager(id, name, gender, department, salary));
break;
case 2:
cout << "请输入技术人员时薪:";
cin >> hourlyWage;
cout << "请输入技术人员工作小时数:";
cin >> workHours;
employees.push_back(new Technician(id, name, gender, department, hourlyWage, workHours));
break;
default:
cout << "无效的选择!" << endl;
return;
}
cout << "添加成功!" << endl;
}
void searchEmployee() {
cout << "请选择查询方式(1.按编号查询 2.按姓名查询 3.显示所有员工):";
int choice;
cin >> choice;
switch (choice) {
case 1:
int id;
cout << "请输入员工编号:";
cin >> id;
Employee* e1 = findEmployeeByID(id);
if (e1 == NULL) {
cout << "未找到该员工!" << endl;
}
else {
e1->printInfo();
}
break;
case 2:
string name;
cout << "请输入员工姓名:";
cin >> name;
vector<Employee*> results = findEmployeesByName(name);
if (results.empty()) {
cout << "未找到该员工!" << endl;
}
else {
for (int i = 0; i < results.size(); i++) {
results[i]->printInfo();
}
}
break;
case 3:
if (employees.empty()) {
cout << "记录为空!" << endl;
}
else {
for (int i = 0; i < employees.size(); i++) {
employees[i]->printInfo();
}
}
break;
default:
cout << "无效的选择!" << endl;
return;
}
}
void editEmployee() {
int id;
cout << "请输入要编辑的员工编号:";
cin >> id;
Employee* e1 = findEmployeeByID(id);
if (e1 == NULL) {
cout << "未找到该员工!" << endl;
return;
}
string name, gender, department;
double salary, hourlyWage, workHours;
cout << "请输入员工姓名(原值为" << e1->getName() << "):";
cin >> name;
cout << "请输入员工性别(原值为" << e1->gender << "):";
cin >> gender;
cout << "请输入员工所在部门(原值为" << e1->department << "):";
cin >> department;
if (dynamic_cast<Manager*>(e1) != NULL) { // 判断员工类型
cout << "请输入经理固定薪水(原值为" << dynamic_cast<Manager*>(e1)->calculateSalary() << "):";
cin >> salary;
dynamic_cast<Manager*>(e1)->Employee::id = id;
dynamic_cast<Manager*>(e1)->Employee::name = name;
dynamic_cast<Manager*>(e1)->Employee::gender = gender;
dynamic_cast<Manager*>(e1)->Employee::department = department;
dynamic_cast<Manager*>(e1)->salary = salary;
}
else if (dynamic_cast<Technician*>(e1) != NULL) {
cout << "请输入技术人员时薪(原值为" << dynamic_cast<Technician*>(e1)->hourlyWage << "):";
cin >> hourlyWage;
cout << "请输入技术人员工作小时数(原值为" << dynamic_cast<Technician*>(e1)->workHours << "):";
cin >> workHours;
dynamic_cast<Technician*>(e1)->Employee::id = id;
dynamic_cast<Technician*>(e1)->Employee::name = name;
dynamic_cast<Technician*>(e1)->Employee::gender = gender;
dynamic_cast<Technician*>(e1)->Employee::department = department;
dynamic_cast<Technician*>(e1)->hourlyWage = hourlyWage;
dynamic_cast<Technician*>(e1)->workHours = workHours;
}
cout << "修改成功!" << endl;
}
void deleteEmployee() {
if (employees.empty()) {
cout << "记录为空!" << endl;
return;
}
int choice;
cout << "请选择删除方式(1.按编号删除 2.按姓名删除):";
cin >> choice;
switch (choice) {
case 1:
int id;
cout << "请输入员工编号:";
cin >> id;
for (vector<Employee*>::iterator it = employees.begin(); it != employees.end(); it++) {
if ((*it)->getID() == id) {
employees.erase(it);
cout << "删除成功!" << endl;
return;
}
}
cout << "未找到该员工!" << endl;
break;
case 2:
string name;
cout << "请输入员工姓名:";
cin >> name;
for (vector<Employee*>::iterator it = employees.begin(); it != employees.end(); it++) {
if ((*it)->getName() == name) {
employees.erase(it);
cout << "删除成功!" << endl;
return;
}
}
cout << "未找到该员工!" << endl;
break;
default:
cout << "无效的选择!" << endl;
return;
}
}
private:
Employee* findEmployeeByID(int id) {
for (int i = 0; i < employees.size(); i++) {
if (employees[i]->getID() == id) {
return employees[i];
}
}
return NULL;
}
vector<Employee*> findEmployeesByName(string name) {
vector<Employee*> results;
for (int i = 0; i < employees.size(); i++) {
if (employees[i]->getName() == name) {
results.push_back(employees[i]);
}
}
return results;
}
void loadEmployees() {
ifstream infile(filename);
if (!infile) { // 如果文件不存在,则创建一个空文件
ofstream outfile(filename);
outfile.close();
return;
}
int id;
string name, gender, department;
double salary, hourlyWage, workHours;
while (infile >> id >> name >> gender >> department >> salary >> hourlyWage >> workHours) {
if (salary != 0) {
employees.push_back(new Manager(id, name, gender, department, salary));
}
else {
employees.push_back(new Technician(id, name, gender, department, hourlyWage, workHours));
}
}
infile.close();
}
void saveEmployees() {
ofstream outfile(filename);
for (int i = 0; i < employees.size(); i++) {
outfile << employees[i]->getID() << " " << employees[i]->getName() << " " << employees[i]->gender << " " << employees[i]->department << " ";
if (dynamic_cast<Manager*>(employees[i]) != NULL) {
outfile << dynamic_cast<Manager*>(employees[i])->calculateSalary() << " 0 0" << endl;
}
else if (dynamic_cast<Technician*>(employees[i]) != NULL) {
outfile << "0 " << dynamic_cast<Technician*>(employees[i])->hourlyWage << " " << dynamic_cast<Technician*>(employees[i])->workHours << endl;
}
}
outfile.close();
}
};
int main() {
SalaryManagementSystem system("employees.txt");
while (true) {
cout << endl;
cout << "请选择操作(1.添加员工 2.查询员工 3.编辑员工 4.删除员工 5.退出):";
int choice;
cin >> choice;
switch (choice) {
case 1:
system.addEmployee();
break;
case 2:
system.searchEmployee();
break;
case 3:
system.editEmployee();
break;
case 4:
system.deleteEmployee();
break;
case 5:
return 0;
default:
cout << "无效的选择!" << endl;
break;
}
}
}
```
注意到代码中使用了虚函数和多态的特性,可以方便地处理不同类型的员工。同时,将员工信息存储到文件中,可以实现程序关闭后数据的持久化,下次运行时可以继续读取之前的员工信息。
相关推荐
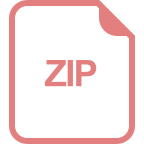
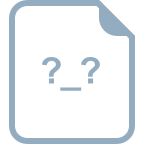













