使用队列来模拟停车场和便道上的车辆,每一次进出车辆都需要对队列进行操作。用C语言实现
时间: 2024-01-21 21:16:17 浏览: 159
以下是一个基本的停车场和便道的队列模拟程序,包括了进入、离开、显示停车场和便道上的车辆等功能。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 5 // 停车场和便道的最大容量
typedef struct {
int id; // 车辆编号
} Car;
typedef struct {
Car data[MAX_SIZE]; // 存储车辆的数组
int front; // 队首指针
int rear; // 队尾指针
} Queue;
void init(Queue *q) {
q->front = 0;
q->rear = 0;
}
int is_empty(Queue *q) {
return q->front == q->rear;
}
int is_full(Queue *q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
void enqueue(Queue *q, Car c) {
if (is_full(q)) {
printf("停车场和便道已满,无法再进入!\n");
return;
}
q->data[q->rear] = c;
q->rear = (q->rear + 1) % MAX_SIZE;
}
Car dequeue(Queue *q) {
if (is_empty(q)) {
printf("停车场和便道已空,无法再离开!\n");
return (Car) {0};
}
Car c = q->data[q->front];
q->front = (q->front + 1) % MAX_SIZE;
return c;
}
void show(Queue *q, const char *name) {
printf("%s上的车辆:\n", name);
if (is_empty(q)) {
printf("无\n");
return;
}
int i;
for (i = q->front; i != q->rear; i = (i + 1) % MAX_SIZE) {
printf("车辆编号:%d\n", q->data[i].id);
}
}
int main() {
Queue parking_lot, driveway;
init(&parking_lot);
init(&driveway);
int choice;
do {
printf("\n");
printf("1. 停车\n");
printf("2. 离开\n");
printf("3. 显示停车场和便道上的车辆\n");
printf("0. 退出\n");
printf("请选择操作:");
scanf("%d", &choice);
switch (choice) {
case 1: {
Car c;
printf("请输入车辆编号:");
scanf("%d", &c.id);
enqueue(&parking_lot, c);
break;
}
case 2: {
dequeue(&parking_lot);
if (!is_empty(&driveway)) {
Car c = dequeue(&driveway);
enqueue(&parking_lot, c);
printf("便道上的车辆已进入停车场!\n");
}
break;
}
case 3: {
show(&parking_lot, "停车场");
show(&driveway, "便道");
break;
}
case 0:
break;
default:
printf("无效操作!\n");
break;
}
} while (choice != 0);
return 0;
}
```
该程序通过定义 `Car` 结构体来存储车辆的编号,定义 `Queue` 结构体来表示队列,实现了队列的基本操作函数(初始化、判断是否为空/满、入队/出队)以及显示队列上的车辆信息的函数。在 `main` 函数中,使用菜单选择的方式来实现停车、离开和显示车辆等操作。其中,当停车场和便道已满时,无法再进入车辆;当停车场和便道已空时,无法再离开车辆。同时,当停车场有空位时,若便道上有等待的车辆,则该车辆将进入停车场。
阅读全文
相关推荐
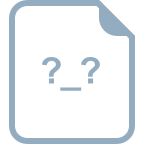
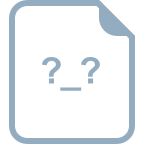
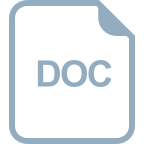
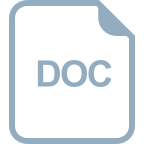
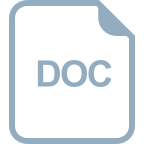









