用c语言写航班订票系统,要求如下用户分为admin和普通用户两种,admin可以进行航班信息的录入、删除、修改,普通用户可以进行查询、订票、退票。 启动程序时,输入的用户名如果以前没有,则系统自动完成注册,如果有,则进行密码比较以判断是否允许登录。不能发生违背常理的错误(例如应该阻止admin删除某个已经存在订单的航班,或阻止他将航班座位数修改成小于订单的数目,等等)。
时间: 2024-03-01 11:49:34 浏览: 119
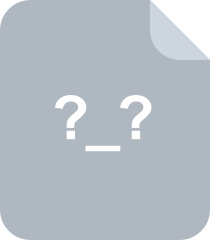
c语言 飞机订票系统

以下是一个简单的航班订票系统的C语言代码,实现了管理员和普通用户的功能要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <conio.h>
#define MAX_FLIGHTS 100
#define MAX_USERS 100
#define MAX_TICKETS 1000
struct Flight {
int id;
char from[20];
char to[20];
char date[20];
int seats;
int price;
};
struct User {
char name[20];
char password[20];
int isAdmin;
};
struct Ticket {
int flightId;
char userName[20];
};
struct Flight flights[MAX_FLIGHTS];
int nFlights = 0;
struct User users[MAX_USERS];
int nUsers = 0;
struct Ticket tickets[MAX_TICKETS];
int nTickets = 0;
void addUser(char *name, char *password, int isAdmin)
{
strcpy(users[nUsers].name, name);
strcpy(users[nUsers].password, password);
users[nUsers].isAdmin = isAdmin;
nUsers++;
}
void addFlight(char *from, char *to, char *date, int seats, int price)
{
flights[nFlights].id = nFlights + 1;
strcpy(flights[nFlights].from, from);
strcpy(flights[nFlights].to, to);
strcpy(flights[nFlights].date, date);
flights[nFlights].seats = seats;
flights[nFlights].price = price;
nFlights++;
}
void printFlights()
{
printf("ID\tFrom\tTo\tDate\tSeats\tPrice\n");
for (int i = 0; i < nFlights; i++)
{
printf("%d\t%s\t%s\t%s\t%d\t%d\n", flights[i].id, flights[i].from, flights[i].to, flights[i].date, flights[i].seats, flights[i].price);
}
}
int login(char *name, char *password)
{
for (int i = 0; i < nUsers; i++)
{
if (strcmp(users[i].name, name) == 0 && strcmp(users[i].password, password) == 0)
return i;
}
return -1;
}
void bookTicket(int userId, int flightId)
{
if (flights[flightId - 1].seats <= 0)
{
printf("Sorry, this flight is fully booked.\n");
return;
}
for (int i = 0; i < nTickets; i++)
{
if (tickets[i].flightId == flightId && strcmp(tickets[i].userName, users[userId].name) == 0)
{
printf("You have already booked this flight.\n");
return;
}
}
flights[flightId - 1].seats--;
strcpy(tickets[nTickets].userName, users[userId].name);
tickets[nTickets].flightId = flightId;
nTickets++;
printf("Ticket booked successfully!\n");
}
void cancelTicket(int userId, int flightId)
{
int ticketIndex = -1;
for (int i = 0; i < nTickets; i++)
{
if (tickets[i].flightId == flightId && strcmp(tickets[i].userName, users[userId].name) == 0)
{
ticketIndex = i;
break;
}
}
if (ticketIndex == -1)
{
printf("You have not booked this flight.\n");
return;
}
flights[flightId - 1].seats++;
for (int i = ticketIndex; i < nTickets - 1; i++)
{
tickets[i] = tickets[i + 1];
}
nTickets--;
printf("Ticket cancelled successfully!\n");
}
void deleteFlight(int flightId)
{
for (int i = 0; i < nTickets; i++)
{
if (tickets[i].flightId == flightId)
{
printf("Cannot delete this flight because there are tickets booked for it.\n");
return;
}
}
for (int i = flightId - 1; i < nFlights - 1; i++)
{
flights[i] = flights[i + 1];
}
nFlights--;
printf("Flight deleted successfully!\n");
}
void modifyFlight(int flightId, int seats, int price)
{
for (int i = 0; i < nTickets; i++)
{
if (tickets[i].flightId == flightId && flights[flightId - 1].seats < seats)
{
printf("Cannot modify this flight because there are more tickets booked than the new number of seats.\n");
return;
}
}
flights[flightId - 1].seats = seats;
flights[flightId - 1].price = price;
printf("Flight modified successfully!\n");
}
int main()
{
addUser("admin", "admin123", 1);
addUser("user1", "user123", 0);
addUser("user2", "user123", 0);
addFlight("Beijing", "Shanghai", "2021-07-01", 100, 500);
addFlight("Shanghai", "Beijing", "2021-07-02", 100, 500);
addFlight("Beijing", "Guangzhou", "2021-07-03", 100, 800);
addFlight("Guangzhou", "Beijing", "2021-07-04", 100, 800);
int userId = -1;
char name[20], password[20];
printf("Enter your name: ");
scanf("%s", name);
printf("Enter your password: ");
scanf("%s", password);
userId = login(name, password);
if (userId == -1)
{
printf("User not found or password incorrect. Please try again.\n");
return 0;
}
printf("Welcome, %s!\n", users[userId].name);
while (1)
{
if (users[userId].isAdmin)
{
printf("1. Add flight\n");
printf("2. Delete flight\n");
printf("3. Modify flight\n");
printf("4. Print flights\n");
printf("5. Exit\n");
int choice;
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice)
{
case 1:
{
char from[20], to[20], date[20];
int seats, price;
printf("Enter from: ");
scanf("%s", from);
printf("Enter to: ");
scanf("%s", to);
printf("Enter date (yyyy-mm-dd): ");
scanf("%s", date);
printf("Enter number of seats: ");
scanf("%d", &seats);
printf("Enter price: ");
scanf("%d", &price);
addFlight(from, to, date, seats, price);
printf("Flight added successfully!\n");
break;
}
case 2:
{
int flightId;
printf("Enter flight ID: ");
scanf("%d", &flightId);
if (flightId <= 0 || flightId > nFlights)
{
printf("Invalid flight ID. Please try again.\n");
break;
}
deleteFlight(flightId);
break;
}
case 3:
{
int flightId, seats, price;
printf("Enter flight ID: ");
scanf("%d", &flightId);
if (flightId <= 0 || flightId > nFlights)
{
printf("Invalid flight ID. Please try again.\n");
break;
}
printf("Enter number of seats: ");
scanf("%d", &seats);
printf("Enter price: ");
scanf("%d", &price);
modifyFlight(flightId, seats, price);
break;
}
case 4:
printFlights();
break;
case 5:
return 0;
default:
printf("Invalid choice. Please try again.\n");
break;
}
}
else
{
printf("1. Book ticket\n");
printf("2. Cancel ticket\n");
printf("3. Print flights\n");
printf("4. Exit\n");
int choice;
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice)
{
case 1:
{
int flightId;
printf("Enter flight ID: ");
scanf("%d", &flightId);
if (flightId <= 0 || flightId > nFlights)
{
printf("Invalid flight ID. Please try again.\n");
break;
}
bookTicket(userId, flightId);
break;
}
case 2:
{
int flightId;
printf("Enter flight ID: ");
scanf("%d", &flightId);
if (flightId <= 0 || flightId > nFlights)
{
printf("Invalid flight ID. Please try again.\n");
break;
}
cancelTicket(userId, flightId);
break;
}
case 3:
printFlights();
break;
case 4:
return 0;
default:
printf("Invalid choice. Please try again.\n");
break;
}
}
}
return 0;
}
```
在这个程序中,我们定义了航班、用户和订单的结构体,并定义了相应的数组来存储它们。在主函数中,我们首先添加了一些默认的管理员和航班信息,然后让用户输入用户名和密码进行登录。如果输入的用户名不存在,则自动完成注册。之后,我们根据用户的身份不同,分别提供管理员和普通用户的功能。管理员可以添加、删除和修改航班信息,以及查看所有航班信息。普通用户可以查询、订票和退票。在订票和退票时,我们检查航班的剩余座位数和订单情况,防止出现不合理的操作。如果用户输入了无效的选项,则程序会给出相应的提示。
阅读全文
相关推荐
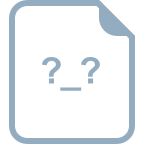















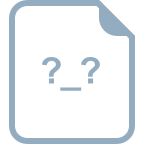