qt 双线性插值算法
时间: 2023-07-13 08:06:36 浏览: 77
双线性插值算法是一种图像缩放算法,可以用于将一张图片放大或缩小到任意大小。下面是使用 Qt 实现双线性插值算法的示例代码:
```cpp
QImage bilinearInterpolation(const QImage& image, int w, int h)
{
QImage result(w, h, QImage::Format_ARGB32);
float scaleX = (float)image.width() / w;
float scaleY = (float)image.height() / h;
for (int y = 0; y < h; y++) {
for (int x = 0; x < w; x++) {
float gx = x * scaleX;
float gy = y * scaleY;
int x1 = (int)gx;
int y1 = (int)gy;
int x2 = x1 + 1;
int y2 = y1 + 1;
if (x2 >= image.width()) x2 = image.width() - 1;
if (y2 >= image.height()) y2 = image.height() - 1;
float fractX = gx - x1;
float fractY = gy - y1;
QColor c11(image.pixel(x1, y1));
QColor c12(image.pixel(x1, y2));
QColor c21(image.pixel(x2, y1));
QColor c22(image.pixel(x2, y2));
float r = (1 - fractX) * (1 - fractY) * c11.redF() + fractX * (1 - fractY) * c21.redF()
+ (1 - fractX) * fractY * c12.redF() + fractX * fractY * c22.redF();
float g = (1 - fractX) * (1 - fractY) * c11.greenF() + fractX * (1 - fractY) * c21.greenF()
+ (1 - fractX) * fractY * c12.greenF() + fractX * fractY * c22.greenF();
float b = (1 - fractX) * (1 - fractY) * c11.blueF() + fractX * (1 - fractY) * c21.blueF()
+ (1 - fractX) * fractY * c12.blueF() + fractX * fractY * c22.blueF();
float a = (1 - fractX) * (1 - fractY) * c11.alphaF() + fractX * (1 - fractY) * c21.alphaF()
+ (1 - fractX) * fractY * c12.alphaF() + fractX * fractY * c22.alphaF();
result.setPixel(x, y, qRgba(r * 255, g * 255, b * 255, a * 255));
}
}
return result;
}
```
这个方法的参数为要缩放的原始图像 `image` 和要生成的缩放后的图像的宽度 `w` 和高度 `h`。算法中使用了 `float` 类型来计算像素坐标和插值系数,所以可以得到更高精度的结果。
相关推荐
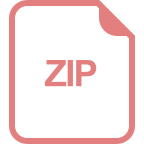














