Import the adjacency matrix of the digraph and output the graph with JavaFX and a graphic user interface. Draw the arrows of the digraph based on the line object of JavaFX. Show me the code by using java language.
时间: 2024-03-19 22:46:13 浏览: 74
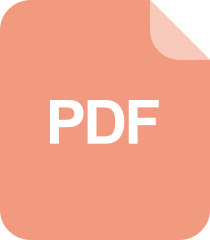
javaFX 入门教程
Sure, here is an example code that imports the adjacency matrix of a digraph, outputs the graph with JavaFX, and draws the arrows of the digraph based on the line object:
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class DigraphGUI extends Application {
// Define the adjacency matrix of the digraph
int[][] adjMatrix = {
{0, 1, 0, 0},
{1, 0, 1, 1},
{0, 0, 0, 1},
{0, 0, 1, 0}
};
// Define the positions of the nodes
int[] nodeX = {50, 150, 250, 350};
int[] nodeY = {50, 150, 150, 250};
@Override
public void start(Stage primaryStage) {
// Create a canvas to draw the graph on
Canvas canvas = new Canvas(400, 300);
GraphicsContext gc = canvas.getGraphicsContext2D();
// Draw the nodes
for (int i = 0; i < adjMatrix.length; i++) {
gc.setFill(Color.BLUEVIOLET);
gc.fillOval(nodeX[i] - 10, nodeY[i] - 10, 20, 20);
gc.setFill(Color.WHITE);
gc.fillText(Integer.toString(i), nodeX[i] - 3, nodeY[i] + 3);
}
// Draw the edges and arrows
for (int i = 0; i < adjMatrix.length; i++) {
for (int j = 0; j < adjMatrix.length; j++) {
if (adjMatrix[i][j] == 1) {
gc.setStroke(Color.BLACK);
gc.setLineWidth(1.5);
gc.strokeLine(nodeX[i], nodeY[i], nodeX[j], nodeY[j]);
double angle = Math.atan2(nodeY[j] - nodeY[i], nodeX[j] - nodeX[i]);
double arrowX = Math.cos(angle - Math.toRadians(20)) * 10 + nodeX[j];
double arrowY = Math.sin(angle - Math.toRadians(20)) * 10 + nodeY[j];
gc.strokeLine(nodeX[j], nodeY[j], arrowX, arrowY);
arrowX = Math.cos(angle + Math.toRadians(20)) * 10 + nodeX[j];
arrowY = Math.sin(angle + Math.toRadians(20)) * 10 + nodeY[j];
gc.strokeLine(nodeX[j], nodeY[j], arrowX, arrowY);
}
}
}
// Create a scene and add the canvas to it
StackPane root = new StackPane();
root.getChildren().add(canvas);
Scene scene = new Scene(root, 400, 300);
// Set the stage and show the scene
primaryStage.setTitle("Digraph GUI");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
In this code, we first define the adjacency matrix of the digraph and the positions of the nodes. Then, we create a canvas and draw the nodes on it. Next, we iterate through the adjacency matrix and draw the edges and arrows based on the line object of JavaFX. Finally, we create a scene and add the canvas to it, then show the scene on the stage.
阅读全文
相关推荐
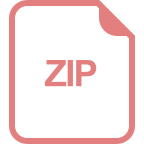














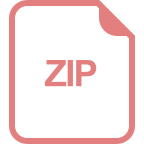
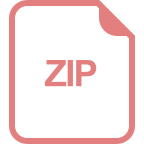
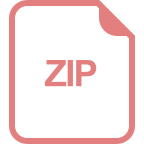
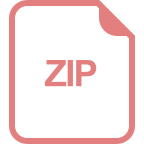