用pyqt5设计神经网络训练GUI界面,并设置红色中止训练按钮
时间: 2024-02-01 08:13:13 浏览: 99
好的,下面是一个简单的例子,用PyQt5实现神经网络训练GUI界面,并设置红色中止训练按钮。
首先,我们需要导入PyQt5库。
```python
from PyQt5.QtWidgets import *
from PyQt5.QtGui import *
from PyQt5.QtCore import *
```
然后,我们创建一个继承自QWidget的类,作为主窗口。
```python
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 设置窗口标题和大小
self.setWindowTitle('神经网络训练GUI界面')
self.setGeometry(100, 100, 800, 600)
# 创建控件
self.input_layer_label = QLabel('输入层:')
self.input_layer_edit = QLineEdit()
self.hidden_layer_label = QLabel('隐藏层:')
self.hidden_layer_edit = QLineEdit()
self.output_layer_label = QLabel('输出层:')
self.output_layer_edit = QLineEdit()
self.train_button = QPushButton('开始训练')
self.stop_button = QPushButton('中止训练')
# 创建布局
grid = QGridLayout()
grid.setSpacing(10)
# 添加控件到布局中
grid.addWidget(self.input_layer_label, 1, 0)
grid.addWidget(self.input_layer_edit, 1, 1)
grid.addWidget(self.hidden_layer_label, 2, 0)
grid.addWidget(self.hidden_layer_edit, 2, 1)
grid.addWidget(self.output_layer_label, 3, 0)
grid.addWidget(self.output_layer_edit, 3, 1)
grid.addWidget(self.train_button, 4, 0)
grid.addWidget(self.stop_button, 4, 1)
# 设置布局
self.setLayout(grid)
# 设置中止训练按钮颜色为红色
self.stop_button.setStyleSheet('background-color: red')
```
在initUI()方法中,我们创建了一些控件,包括三个QLabel、三个QLineEdit、一个QPushButton和一个红色的QPushButton,并将它们添加到一个QGridLayout布局中,最后将该布局设置为主窗口的布局。
最后一行代码设置中止训练按钮的背景颜色为红色。
接下来,我们创建一个QApplication对象,并将主窗口显示出来。
```python
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
完整代码如下:
```python
import sys
from PyQt5.QtWidgets import *
from PyQt5.QtGui import *
from PyQt5.QtCore import *
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 设置窗口标题和大小
self.setWindowTitle('神经网络训练GUI界面')
self.setGeometry(100, 100, 800, 600)
# 创建控件
self.input_layer_label = QLabel('输入层:')
self.input_layer_edit = QLineEdit()
self.hidden_layer_label = QLabel('隐藏层:')
self.hidden_layer_edit = QLineEdit()
self.output_layer_label = QLabel('输出层:')
self.output_layer_edit = QLineEdit()
self.train_button = QPushButton('开始训练')
self.stop_button = QPushButton('中止训练')
# 创建布局
grid = QGridLayout()
grid.setSpacing(10)
# 添加控件到布局中
grid.addWidget(self.input_layer_label, 1, 0)
grid.addWidget(self.input_layer_edit, 1, 1)
grid.addWidget(self.hidden_layer_label, 2, 0)
grid.addWidget(self.hidden_layer_edit, 2, 1)
grid.addWidget(self.output_layer_label, 3, 0)
grid.addWidget(self.output_layer_edit, 3, 1)
grid.addWidget(self.train_button, 4, 0)
grid.addWidget(self.stop_button, 4, 1)
# 设置布局
self.setLayout(grid)
# 设置中止训练按钮颜色为红色
self.stop_button.setStyleSheet('background-color: red')
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
阅读全文
相关推荐
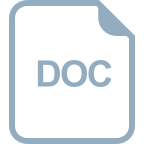
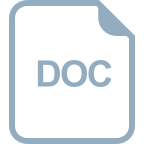
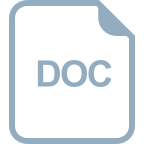


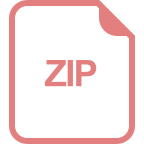
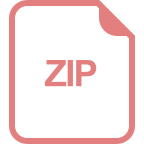
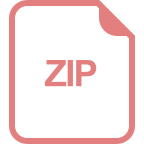
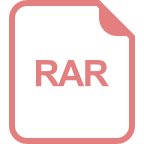
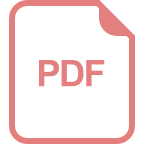
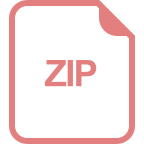
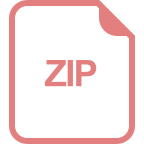
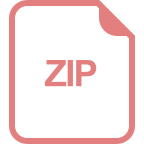
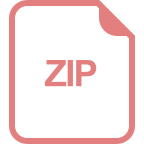
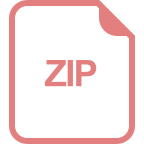