A* Pathfinding Project插件中写一个脚本: 需求:1.每帧获取当前位置到达终点路径数据,并添加终点vector3到路径数组的最后位置,脚本中创建一个路径数组LastPath,用于记录最后一次路径信息,对比当前路径与LastPath是否相同,相同则逻辑停止,否则把LastPath替换为当前路径,根据这个路径数组在场景中使用linerender渲染出一条路径,全局只有一个linerender
时间: 2023-12-10 15:41:32 浏览: 29
好的,可以按照以下步骤实现该脚本:
1. 创建一个新的C#脚本,并将其命名为AStarPathfinding。
2. 在脚本中定义一个路径数组LastPath,用于记录最后一次路径信息。同时定义一个bool类型的变量isPathfinding,用于表示当前是否正在搜索路径。
3. 在Start()方法中获取场景中的Linerenderer组件,并将其保存在一个变量中,以便在后续方法中使用。
4. 在Update()方法中,首先判断isPathfinding是否为false,如果是,则调用A*算法搜索路径,并将isPathfinding设置为true。搜索路径的方法可以根据你的具体需求自己实现,这里不再详细介绍。
5. 如果搜索路径成功,将获取的路径数据存储在一个新的路径数组中,然后将终点vector3添加到该路径数组的最后一个位置。
6. 接下来,比较当前路径数组与LastPath是否相同。如果相同,则不做任何操作,直接返回。如果不同,则将当前路径数组赋值给LastPath,然后使用Linerenderer组件在场景中渲染路径。
7. 最后,在OnDestroy()方法中销毁Linerenderer组件。
完整代码如下所示:
```
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class AStarPathfinding : MonoBehaviour
{
private LineRenderer lineRenderer; // 用于渲染路径的Linerenderer组件
private List<Vector3> LastPath = new List<Vector3>(); // 记录最后一次路径信息的路径数组
private bool isPathfinding = false; // 是否正在搜索路径的标志位
void Start()
{
// 获取Linerenderer组件
lineRenderer = GetComponent<LineRenderer>();
}
void Update()
{
if (!isPathfinding)
{
// 调用A*算法搜索路径
List<Vector3> path = FindPath();
if (path != null)
{
// 将终点vector3添加到路径数组中
path.Add(transform.position);
// 判断当前路径是否与上一次路径相同
if (!IsSamePath(path))
{
// 更新LastPath
LastPath = path;
// 使用Linerenderer渲染路径
RenderPath();
}
}
// 将isPathfinding设置为false,表示路径搜索结束
isPathfinding = false;
}
}
// 使用A*算法搜索路径的方法,具体实现可以根据需求自己编写
List<Vector3> FindPath()
{
// TODO
return null;
}
// 判断当前路径与上一次路径是否相同的方法
bool IsSamePath(List<Vector3> path)
{
if (LastPath.Count != path.Count)
{
return false;
}
for (int i = 0; i < LastPath.Count; i++)
{
if (LastPath[i] != path[i])
{
return false;
}
}
return true;
}
// 使用Linerenderer组件渲染路径的方法
void RenderPath()
{
lineRenderer.positionCount = LastPath.Count;
for (int i = 0; i < LastPath.Count; i++)
{
lineRenderer.SetPosition(i, LastPath[i]);
}
}
void OnDestroy()
{
// 销毁Linerenderer组件
Destroy(lineRenderer);
}
}
```
相关推荐
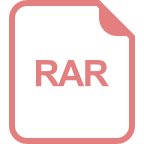
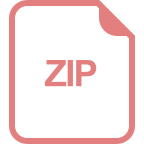
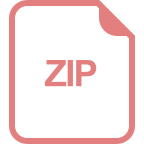














