class SelfAttention(nn.Module): def __init__(self, in_channels, reduction=4): super(SelfAttention, self).__init__() self.avg_pool = nn.AdaptiveAvgPool2d((1, 1)) self.fc1 = nn.Conv2d(in_channels, in_channels // reduction, 1, bias=False) self.relu = nn.ReLU(inplace=True) self.fc2 = nn.Conv2d(in_channels // reduction, in_channels, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): # print("x=", x) b, c, n = x.size() y = self.avg_pool(x) y = self.fc1(y) y = self.relu(y) y = self.fc2(y) y = self.sigmoid(y) return x * y.expand_as(x)这是我注意力机制模块的代码,却在运行时报错提示: File "/root/autodl-tmp/project/tools/../lib/net/pointnet2_msg.py", line 91, in forward y = self.fc1(y) File "/root/miniconda3/lib/python3.8/site-packages/torch/nn/modules/module.py", line 727, in _call_impl result = self.forward(*input, **kwargs) File "/root/miniconda3/lib/python3.8/site-packages/torch/nn/modules/conv.py", line 423, in forward return self._conv_forward(input, self.weight) File "/root/miniconda3/lib/python3.8/site-packages/torch/nn/modules/conv.py", line 419, in _conv_forward return F.conv2d(input, weight, self.bias, self.stride, RuntimeError: Expected 4-dimensional input for 4-dimensional weight [256, 1024, 1, 1], but got 3-dimensional input of size [16, 1, 1] instead
时间: 2023-11-19 14:05:56 浏览: 186
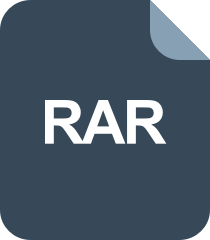
PAPR.rar_PAPR_in_ofdm papr_ofdm papr reduction_papr reduction

这个错误是由于你的输入张量 `x` 的维度不正确,期望是一个 4 维张量,但是实际上 `x` 是一个 3 维张量。可以看出,你的 `x` 的尺寸为 `[batch_size, channel, n]`,其中 `n` 表示序列的长度,并且你使用了全局平均池化层对 `n` 进行了降维。最终,`y` 的尺寸应该是 `[batch_size, channel // reduction, 1, 1]`。但是,在你使用 `self.fc1(y)` 进行卷积时,它期望 `y` 是一个 4 维张量,而实际上 `y` 是一个 3 维张量,所以会报错。
解决这个问题,你可以在 `forward` 函数中增加一个维度,将 `y` 变成一个 4 维张量。修改后的代码如下:
```
class SelfAttention(nn.Module):
def __init__(self, in_channels, reduction=4):
super(SelfAttention, self).__init__()
self.avg_pool = nn.AdaptiveAvgPool2d((1, 1))
self.fc1 = nn.Conv2d(in_channels, in_channels // reduction, 1, bias=False)
self.relu = nn.ReLU(inplace=True)
self.fc2 = nn.Conv2d(in_channels // reduction, in_channels, 1, bias=False)
self.sigmoid = nn.Sigmoid()
def forward(self, x):
b, c, n = x.size()
y = self.avg_pool(x)
y = y.unsqueeze(-1).unsqueeze(-1) # 增加两个维度,变成 4 维张量
y = self.fc1(y)
y = self.relu(y)
y = self.fc2(y)
y = self.sigmoid(y)
return x * y.expand_as(x)
```
增加维度的操作使用 `unsqueeze` 函数,将 `y` 在最后两个维度上增加维度。这样就可以避免报错了。
阅读全文