分别用文本文件和CSV文件保存5个学生的课程成绩。编写一个程序,从文件读取成绩,计算总分,按总分按从高到低的顺序输出。
时间: 2024-02-22 14:57:41 浏览: 83
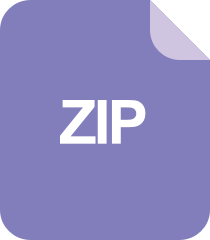
IPL:该程序读取csv文件并在控制台中打印
以下是Python代码示例:
使用文本文件保存成绩:
```python
# 读取文本文件
with open('scores.txt', 'r') as f:
lines = f.readlines()
scores = {}
# 解析数据
for line in lines:
items = line.strip().split(',')
name = items[0]
score = list(map(int, items[1:]))
scores[name] = score
# 计算总分
total_scores = {}
for name, score in scores.items():
total_scores[name] = sum(score)
# 排序并输出
sorted_scores = sorted(total_scores.items(), key=lambda x: x[1], reverse=True)
for name, total_score in sorted_scores:
print(f'{name}: {total_score}')
```
使用CSV文件保存成绩:
```python
import csv
# 读取CSV文件
with open('scores.csv', 'r') as f:
reader = csv.reader(f)
header = next(reader)
scores = {}
for row in reader:
name = row[0]
score = list(map(int, row[1:]))
scores[name] = score
# 计算总分
total_scores = {}
for name, score in scores.items():
total_scores[name] = sum(score)
# 排序并输出
sorted_scores = sorted(total_scores.items(), key=lambda x: x[1], reverse=True)
for name, total_score in sorted_scores:
print(f'{name}: {total_score}')
```
注意:在使用CSV文件时,需要使用Python标准库中的csv模块来读取文件。并且,第一行通常是标题行,需要跳过。
阅读全文
相关推荐
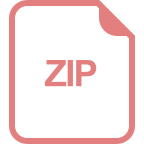
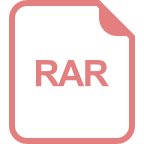














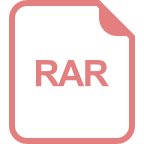