int main() { char str[3][80]; int upper = 0, lower = 0, chinese = 0, digit = 0, space = 0, others = 0; int i, j; for (i = 0; i < 3; i++) { gets(str[i]); for (j = 0; j < 80 && str[i][j] != '\0'; j++) { if (str[i][j] >= 'A' && str[i][j] <= 'Z') { upper++; } else if (str[i][j] >= 'a' && str[i][j] <= 'z') { lower++; } else if (str[i][j] >= '0' && str[i][j] <= '9') { digit++; } else if (str[i][j] == ' ') { space++; } else if (str[i][j] >= 128) { chinese++; } else { others++; } } } printf("upper:%d\n", upper); printf("lower:%d\n", lower); printf("chinese:%d\n", chinese/3); printf("digit:%d\n", digit); printf("space:%d\n", space); printf("others:%d\n", others); return 0; }
时间: 2023-06-23 17:10:27 浏览: 140
这是一个统计输入的三行字符串中各种字符出现次数的程序。代码中定义了一个字符数组 `str`,用来存储输入的三行字符串;以及六个变量 `upper`、`lower`、`chinese`、`digit`、`space`、`others`,分别用来存储大写字母、小写字母、中文字符、数字、空格和其他字符的出现次数。
程序使用了两层循环,外层循环控制输入三行字符串的过程,内层循环遍历每个字符串的每个字符,根据字符的种类更新相应计数器的值。具体而言,如果是大写字母,则 `upper` 计数器加一;如果是小写字母,则 `lower` 计数器加一;如果是数字,则 `digit` 计数器加一;如果是空格,则 `space` 计数器加一;如果是非 ASCII 字符,则 `chinese` 计数器加一;否则,说明是其他字符,`others` 计数器加一。
最后输出各种字符出现的次数即可。需要注意的是,由于输入的三行字符串长度不一定相等,因此内层循环需要在判断字符不为 '\0' 时终止。另外,中文字符的判断使用了一个简单的近似方法,即将中文字符的数量除以 3,这是因为中文字符在 ASCII 编码中占据了三个字节。
相关问题
程序改错 功能:输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数。 #include <stdio.h> void main(void) { char str[80]; int i; int letters=0,space=0,digit=0,others=0; printf("please input a string:\n"); get(str); for(i=0;str[i]!='\0';i++) { if(str[i]>='a'&&str[i]<=z'&&str[j]>='A'&&str[i]<=Z) letters++; else if(str[i]=!'") space++; else if(str[i]>='0'&&str[i]<='9') digit++; else others++; } printf("char=%d space=%d digit=%d others=%d\n",letters,space,digit,others); }
程序有以下几处错误:
1. `get()` 函数应该改为 `gets()` 函数。
2. 第10行的 `str[j]` 应该改为 `str[i]`。
3. 第12行的判断条件应该是 `str[i]!=' ' && str[i]!= '\t'`,即判断字符不是空格也不是制表符。
4. 第13行的判断条件应该是 `str[i]>='0'&&str[i]<='9'`,即判断字符是数字。
修改后的程序如下所示:
```c
#include <stdio.h>
void main(void) {
char str[80];
int i;
int letters=0,space=0,digit=0,others=0;
printf("please input a string:\n");
gets(str);
for(i=0;str[i]!='\0';i++) {
if((str[i]>='a'&&str[i]<='z')||(str[i]>='A'&&str[i]<='Z'))
letters++;
else if(str[i]!=' ' && str[i]!= '\t')
space++;
else if(str[i]>='0'&&str[i]<='9')
digit++;
else
others++;
}
printf("char=%d space=%d digit=%d others=%d\n",letters,space,digit,others);
}
```
def get_strong _password_state ( pwd ) : count_all = len ( pwd) count_upper = o count_lower = 0count_digit = 0for char in pwd: if char.isupper ( ) : count_upper += 1elif char.islower ( ) : count__lower += 1elif char .isdigit ( ) : count_digit += 1 #请完善此处代
这是一个 Python 函数,名为 get_strong_password_state,用于检查密码强度。它接受一个字符串参数 pwd,代表密码。函数会统计密码中包含的字符数量,并分别计算大写字母、小写字母和数字的数量。如果密码中包含大写字母,则 count_upper 变量加一;如果密码中包含小写字母,则 count_lower 变量加一;如果密码中包含数字,则 count_digit 变量加一。最终函数会返回一个包含这三个变量的元组,代表密码的强度状态。
阅读全文
相关推荐
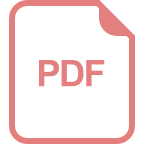
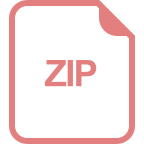
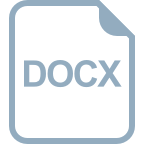














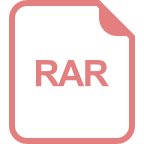