用c语言写出贪吃蛇小游戏
时间: 2023-12-06 21:03:59 浏览: 96
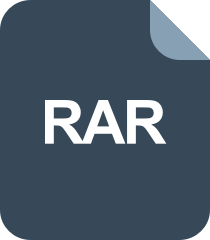
tanchishe.rar_c 小游戏代码_c语言 小游戏_c语言 贪吃蛇_c语言小游戏_贪吃蛇
好的,以下是一个简单的贪吃蛇小游戏的 C 语言代码:
```
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <time.h>
#define MAP_WIDTH 20
#define MAP_HEIGHT 20
#define SNAKE_MAX_LENGTH 100
int map[MAP_WIDTH][MAP_HEIGHT];
int snakeX[SNAKE_MAX_LENGTH], snakeY[SNAKE_MAX_LENGTH];
int snakeLength = 0;
int score = 0;
int foodX = 0, foodY = 0;
int direction = 1; // 1: up, 2: right, 3: down, 4: left
void initMap() {
for (int i = 0; i < MAP_WIDTH; i++) {
for (int j = 0; j < MAP_HEIGHT; j++) {
if (i == 0 || i == MAP_WIDTH - 1 || j == 0 || j == MAP_HEIGHT - 1) {
map[i][j] = 1;
} else {
map[i][j] = 0;
}
}
}
}
void printMap() {
system("cls");
for (int i = 0; i < MAP_WIDTH; i++) {
for (int j = 0; j < MAP_HEIGHT; j++) {
if (map[i][j] == 1) {
printf("#");
} else if (map[i][j] == 2) {
printf("*");
} else if (map[i][j] == 3) {
printf("O");
} else {
printf(" ");
}
}
printf("\n");
}
printf("Score: %d\n", score);
}
void generateFood() {
srand((unsigned int)time(NULL));
int x, y;
do {
x = rand() % (MAP_WIDTH - 2) + 1;
y = rand() % (MAP_HEIGHT - 2) + 1;
} while (map[x][y] != 0);
foodX = x;
foodY = y;
map[foodX][foodY] = 2;
}
void initSnake() {
int x = MAP_WIDTH / 2, y = MAP_HEIGHT / 2;
snakeX[0] = x;
snakeY[0] = y;
snakeLength = 1;
map[x][y] = 3;
}
void updateSnake() {
int x = snakeX[0], y = snakeY[0];
switch (direction) {
case 1: y--; break;
case 2: x++; break;
case 3: y++; break;
case 4: x--; break;
}
if (x == foodX && y == foodY) {
snakeLength++;
score += 10;
generateFood();
} else {
int tailX = snakeX[snakeLength - 1], tailY = snakeY[snakeLength - 1];
map[tailX][tailY] = 0;
for (int i = snakeLength - 1; i > 0; i--) {
snakeX[i] = snakeX[i - 1];
snakeY[i] = snakeY[i - 1];
}
snakeX[0] = x;
snakeY[0] = y;
}
if (map[x][y] == 1 || map[x][y] == 3) {
printf("Game over!\n");
exit(0);
}
map[x][y] = 3;
}
void handleInput() {
if (_kbhit()) {
int ch = _getch();
if (ch == 'w' || ch == 'W') {
if (direction != 3) {
direction = 1;
}
} else if (ch == 'd' || ch == 'D') {
if (direction != 4) {
direction = 2;
}
} else if (ch == 's' || ch == 'S') {
if (direction != 1) {
direction = 3;
}
} else if (ch == 'a' || ch == 'A') {
if (direction != 2) {
direction = 4;
}
}
}
}
int main() {
initMap();
generateFood();
initSnake();
while (1) {
printMap();
updateSnake();
handleInput();
Sleep(100);
}
return 0;
}
```
希望能帮到你!
阅读全文
相关推荐
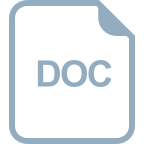
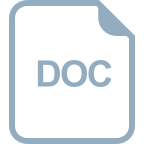

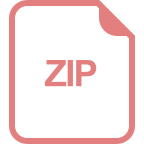
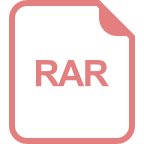




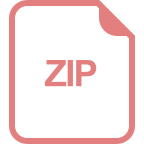
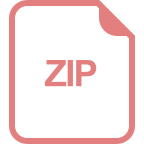
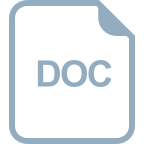





