请用c语言写一个贪吃蛇
时间: 2023-08-31 22:26:34 浏览: 53
### 回答1:
好的,我来用C语言写一个“贪吃蛇”的小游戏。
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#define WIDTH 20
#define HEIGHT 20
int map[WIDTH][HEIGHT] = { 0 }; // 游戏地图
int snake[WIDTH*HEIGHT][2]; // 蛇的位置
int snakeLength = 3; // 蛇的长度
int dir[2] = { 0, 1 }; // 蛇的移动方向
void drawMap() // 画出游戏地图和蛇的位置
{
system("cls"); // 清空屏幕
// 画出游戏地图
for (int i = 0; i < WIDTH + 2; i++) {
printf("#");
}
printf("\n");
for (int i = 0; i < HEIGHT; i++) {
printf("#");
for (int j = 0; j < WIDTH; j++) {
if (map[j][i] == 0) {
printf(" ");
}
else if (map[j][i] == 1) {
printf("*");
}
else if (map[j][i] == 2) {
printf("O");
}
}
printf("#\n");
}
for (int i = 0; i < WIDTH + 2; i++) {
printf("#");
}
printf("\n");
// 画出蛇的位置
for (int i = 0; i < snakeLength; i++) {
printf("%d, %d\n", snake[i][0], snake[i][1]);
}
}
void initSnake() // 初始化蛇的位置
{
int x = WIDTH / 2;
int y = HEIGHT / 2;
snake[0][0] = x;
snake[0][1] = y;
map[x][y] = 2;
for (int i = 1; i < snakeLength; i++) {
snake[i][0] = x - i;
snake[i][1] = y;
map[x - i][y] = 1;
}
}
void moveSnake() // 移动蛇的位置
{
int x = snake[0][0] + dir[0];
int y = snake[0][1] + dir[1];
if (x < 0 || x >= WIDTH || y < 0 || y >= HEIGHT) { // 蛇撞墙了
exit(0);
}
if (map[x][y] == 1) { // 蛇撞到自己了
exit(0);
}
if (map[x][y] == 0) { // 蛇移动到空地上
map[snake[snakeLength - 1][0]][snake[snakeLength - 1][1]] = 0;
for (int i = snakeLength - 1; i > 0; i--) {
snake[i][0] = snake[i - 1][0];
snake[i][1] = snake[i - 1][1];
}
snake[0][0] = x;
snake[0][1] = y;
map[x][y] = 2;
### 回答2:
贪吃蛇是一款经典的游戏,通过操纵蛇的移动吃食物,并随着吃食物的增加身体变长,直到吃到自己的身体或者撞到墙壁游戏结束。下面是一个用C语言实现的简易贪吃蛇游戏代码示例:
#include <stdio.h>
#include <conio.h>
#include <windows.h>
#define UP 72
#define DOWN 80
#define LEFT 75
#define RIGHT 77
int length = 3;
int bend_no;
int len;
char key;
void gotoxy(int x, int y)
{
COORD coord;
coord.X = x;
coord.Y = y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), coord);
}
void bend()
{
int i, j, k;
if (len == length)
{
for (i = bend_no; i > 0; i--)
{
b[i].x = b[i - 1].x;
b[i].y = b[i - 1].y;
}
if (key == RIGHT)
{
b[0].x++;
}
if (key == LEFT)
{
b[0].x--;
}
if (key == UP)
{
b[0].y--;
}
if (key == DOWN)
{
b[0].y++;
}
if (b[0].x > 69)
{
b[0].x = 0;
}
if (b[0].x < 0)
{
b[0].x = 69;
}
if (b[0].y < 0)
{
b[0].y = 19;
}
if (b[0].y > 19)
{
b[0].y = 0;
}
for (i = 0; i < bend_no; i++)
{
if (b[i].x == b[0].x && b[i].y == b[0].y)
{
gameOver = 1;
}
}
if (!gameOver)
{
gotoxy(b[0].x, b[0].y);
printf("O");
}
}
}
void Board()
{
system("cls");
int i, j;
for (i = 0; i <= 20; i++)
{
for (j = 0; j <= 70; j++)
{
if (i == 0 || i == 20 || j == 0 || j == 70)
{
printf("#");
}
else
{
printf(" ");
}
}
printf("\n");
}
printf("Score: %d", length - 1);
printf("\n");
printf("Press X to quit the game");
}
void Food()
{
if (b[0].x == food.x && b[0].y == food.y)
{
score++;
length++;
if (length % 5 == 0)
{
delay--;
}
time_t a;
srand((unsigned)&a);
food.x = rand() % 20;
if (food.x == 0)
{
food.x = 10;
}
food.y = rand() % 20;
if (food.y == 0)
{
food.y = 10;
}
}
else if (food.x == 0)
{
food.x = 10;
food.y = 10;
}
}
void Move()
{
int i;
do
{
Food();
fflush(stdin);
len = 0;
for (i = 0; i < 30; i++)
{
b[i].x = 0;
b[i].y = 0;
if (i == length)
{
break;
}
}
Board();
if (kbhit())
{
key = getch();
if (key == 'x' || key == 'X')
{
gameOver = 1;
break;
}
}
bend();
if (!gameOver)
{
gotoxy(food.x, food.y);
printf("*");
}
delay(delay);
}
while (!gameOver);
}
int main()
{
char key;
Print();
system("cls");
Move();
return 0;
}
### 回答3:
贪吃蛇是一款经典的游戏,可以用C语言来实现。下面是一个简单的贪吃蛇游戏的实现代码:
```c
#include <stdio.h>
#include <conio.h>
#include <windows.h>
// 定义地图的大小
#define WIDTH 20
#define HEIGHT 20
// 定义蛇的最大长度
#define MAX_LENGTH 100
// 定义蛇的方向
enum Direction { UP, DOWN, LEFT, RIGHT };
// 定义蛇的结构体
struct Snake {
int x, y; // 蛇头坐标
int length; // 蛇的长度
int bodyX[MAX_LENGTH], bodyY[MAX_LENGTH]; // 蛇的身体坐标
enum Direction dir; // 蛇的方向
};
// 定义食物的结构体
struct Food {
int x, y; // 食物坐标
};
struct Snake snake; // 创建一个蛇
struct Food food; // 创建一个食物
// 初始化游戏
void initGame() {
// 初始化蛇的位置和长度
snake.x = WIDTH / 2;
snake.y = HEIGHT / 2;
snake.length = 1;
snake.bodyX[0] = snake.x;
snake.bodyY[0] = snake.y;
snake.dir = RIGHT;
// 初始化食物的位置
food.x = rand() % WIDTH;
food.y = rand() % HEIGHT;
}
// 绘制地图和蛇、食物
void drawMap() {
system("cls"); // 清屏
for (int i = 0; i < HEIGHT + 2; i++) {
for (int j = 0; j < WIDTH + 2; j++) {
if (i == 0 || i == HEIGHT + 1 || j == 0 || j == WIDTH + 1) {
printf("#"); // 绘制地图边界
} else if (i == food.y && j == food.x) {
printf("*"); // 绘制食物
} else if (i == snake.y && j == snake.x) {
printf("@"); // 绘制蛇头
} else {
int isBody = 0;
for (int k = 0; k < snake.length; k++) {
if (i == snake.bodyY[k] && j == snake.bodyX[k]) {
printf("#"); // 绘制蛇身
isBody = 1;
break;
}
}
if (!isBody) {
printf(" ");
}
}
}
printf("\n");
}
}
// 蛇移动
void move() {
for (int i = snake.length - 1; i > 0; i--) {
snake.bodyX[i] = snake.bodyX[i - 1];
snake.bodyY[i] = snake.bodyY[i - 1];
}
snake.bodyX[0] = snake.x;
snake.bodyY[0] = snake.y;
switch (snake.dir) {
case UP:
snake.y--;
break;
case DOWN:
snake.y++;
break;
case LEFT:
snake.x--;
break;
case RIGHT:
snake.x++;
break;
}
}
// 处理键盘输入
void handleInput() {
if (_kbhit()) {
switch (_getch()) {
case 'w':
snake.dir = UP;
break;
case 's':
snake.dir = DOWN;
break;
case 'a':
snake.dir = LEFT;
break;
case 'd':
snake.dir = RIGHT;
break;
}
}
}
// 判断游戏结束的条件
int isGameOver() {
if (snake.x < 1 || snake.x > WIDTH || snake.y < 1 || snake.y > HEIGHT) {
return 1; // 碰到地图边界,游戏结束
}
for (int i = 1; i < snake.length; i++) {
if (snake.x == snake.bodyX[i] && snake.y == snake.bodyY[i]) {
return 1; // 碰到自己的身体,游戏结束
}
}
return 0;
}
// 更新游戏状态
void updateGame() {
if (snake.x == food.x && snake.y == food.y) {
// 吃到食物,长度增加,重新生成下一个食物
snake.length++;
food.x = rand() % WIDTH;
food.y = rand() % HEIGHT;
}
}
int main() {
initGame(); // 初始化游戏
while (1) {
drawMap(); // 绘制地图
handleInput(); // 处理键盘输入
move(); // 蛇移动
updateGame(); // 更新游戏状态
if (isGameOver()) {
printf("Game Over!\n");
break;
}
Sleep(200); // 控制游戏速度
}
return 0;
}
```
这是一个比较简单的贪吃蛇游戏实现,有一些基本的功能,包括:
1. 蛇的初始化
2. 地图和蛇、食物的绘制
3. 蛇的移动和键盘输入的处理
4. 判断游戏结束的条件
5. 更新游戏状态
其中,使用了`conio.h`头文件来实现键盘输入的处理,使用了`windows.h`头文件中的`Sleep()`函数来控制游戏的速度。
相关推荐
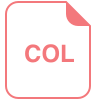
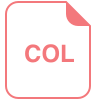
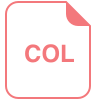
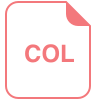
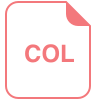









