id_type = random.choice(["身份证", "护照"]) # 证件号 id_number = ''.join(str(random.randint(0, 9)) for _ in range(18)) if id_type == "身份证" else ''.join(str(random.randint(0, 9)) for _ in range(9))
时间: 2024-04-06 07:28:12 浏览: 108
这段代码的作用是生成一个随机的证件类型和号码。其中,变量id_type被赋值为列表["身份证", "护照"]中的一个元素,即随机选择一种证件类型。变量id_number则根据id_type的值生成相应长度的证件号。如果id_type等于"身份证",则生成一个18位的随机数字字符串;否则,生成一个9位的随机数字字符串。
相关问题
优化:def generate_data(add_user): # 学/工号 id_num = random.randint(100000, 999999) # 姓名 first_names = ['张', '王', '李', '刘', '赵', '陈', '周', '钱', "孙", "吴", "曾", "冯", "陶", "将", "黄", "胡", "朱", "杨", "江", "何"] s_names = ['云', '风', '山', '河', '林', '羽', '行', "雪", '月', '雨', '冰', '雷', '鞥', '时', '文', '代', '格', '台', "发", '方', '欧', '白'] l_names = ['雷', '鞥', '时', '文', '代', '格', '台', "发", '方', '欧', '白'] name = random.choice(first_names) + random.choice(s_names) + random.choice(l_names) # 性别 gender = random.choice(['男', '女']) # 身份 identity = random.choice(["学生"]) # 部门 department = random.choice( ["20230608094700005793941100036910"]) # 手机号 phone_num = '1' + str(random.randint(3, 9)) + ''.join(str(random.randint(0, 9)) for _ in range(9)) id_types = ['身份证', '护照', '驾驶证', '学生证', '工作证'] if add_user: id_type = random.choice(id_types) if id_type == '身份证': id_num = ''.join(str(random.randint(0, 9)) for _ in range(18)) elif id_type == '护照': id_num = ''.join(str(random.randint(0, 9)) for _ in range(9)) elif id_type == '驾驶证': id_num = ''.join(str(random.randint(0, 9)) for _ in range(12)) elif id_type == '学生证': id_num = ''.join(str(random.randint(0, 9)) for _ in range(10)) else: id_num = ''.join(str(random.randint(0, 9)) for _ in range(8)) return [id_num, name, gender, phone_num, identity, department, phone_num, id_type, id_num] else: return [name, gender, identity, department, phone_num]
以下是对该函数的一些优化建议:
1. 减少重复代码:函数中有两行代码都生成了手机号,可以将其中一行删掉。
2. 函数参数命名:`add_user` 这个参数名不太符合语义,建议改成 `include_id_num` 或类似的名称。
3. 减少代码行数:可以将 `id_num` 的生成放到 `if add_user:` 的条件判断中,从而减少重复代码。
4. 使用 f-string:可以使用 f-string 来简化字符串拼接。
5. 使用列表推导式:可以使用列表推导式来生成 `first_names`、`s_names` 和 `l_names` 列表。
下面是修改后的代码:
```python
import random
def generate_data(include_id_num):
# 姓名
first_names = ['张', '王', '李', '刘', '赵', '陈', '周', '钱', "孙", "吴", "曾", "冯", "陶", "将", "黄", "胡", "朱", "杨", "江", "何"]
s_names = ['云', '风', '山', '河', '林', '羽', '行', "雪", '月', '雨', '冰', '雷', '鞥', '时', '文', '代', '格', '台', "发", '方', '欧', '白']
l_names = ['雷', '鞥', '时', '文', '代', '格', '台', "发", '方', '欧', '白']
name = random.choice(first_names) + random.choice(s_names) + random.choice(l_names)
# 性别
gender = random.choice(['男', '女'])
# 身份
identity = random.choice(["学生"])
# 部门
department = random.choice(["20230608094700005793941100036910"])
# 手机号
phone_num = '1' + str(random.randint(3, 9)) + ''.join(str(random.randint(0, 9)) for _ in range(9))
if include_id_num:
# 学/工号
id_num = random.randint(100000, 999999)
# 证件类型和号码
id_types = ['身份证', '护照', '驾驶证', '学生证', '工作证']
id_type = random.choice(id_types)
if id_type == '身份证':
id_num = ''.join(str(random.randint(0, 9)) for _ in range(18))
elif id_type == '护照':
id_num = ''.join(str(random.randint(0, 9)) for _ in range(9))
elif id_type == '驾驶证':
id_num = ''.join(str(random.randint(0, 9)) for _ in range(12))
elif id_type == '学生证':
id_num = ''.join(str(random.randint(0, 9)) for _ in range(10))
else:
id_num = ''.join(str(random.randint(0, 9)) for _ in range(8))
return [str(id_num), name, gender, phone_num, identity, department, id_type, id_num, phone_num]
else:
return [name, gender, identity, department, phone_num]
```
将这段代码降低重复率:def muSol(model): sol_list=copy.deepcopy(model.sol_list)#先将初始种群进行复制,然后清空,方便填入新个体 model.sol_list=[] while True: f1_index = int(random.randint(0, len(sol_list) - 1))#在种群中随机选择一个索引 f1 = copy.deepcopy(sol_list[f1_index])#将索引对应的个体深拷贝 m1_index=random.randint(0,len(model.demand_id_list)-1)#随机产生个体的两个索引 m2_index=random.randint(0,len(model.demand_id_list)-1) if m1_index!=m2_index:#如果两个索引不同,则产生一个随机数小于变异率的话将两个索引对应的值进行交换操作 if random.random() <= model.pm: node1=f1.node_id_list[m1_index] f1.node_id_list[m1_index]=f1.node_id_list[m2_index] f1.node_id_list[m2_index]=node1 model.sol_list.append(copy.deepcopy(f1)) else:#如果相同,则把f1这个个体深拷贝到新种群中 model.sol_list.append(copy.deepcopy(f1)) if len(model.sol_list)>model.popsize:#直到新种群中个体数量超过popsize(种群规模) break
可以使用函数参数和内部函数的方式来降低代码重复率,重构后的代码如下:
```python
import copy
import random
def muSol(model):
def swap_nodes(f1, m1_index, m2_index):
# 如果两个索引不同,则产生一个随机数小于变异率的话将两个索引对应的值进行交换操作
if random.random() <= model.pm:
node1 = f1.node_id_list[m1_index]
f1.node_id_list[m1_index] = f1.node_id_list[m2_index]
f1.node_id_list[m2_index] = node1
model.sol_list.append(copy.deepcopy(f1))
else:
# 如果相同,则把f1这个个体深拷贝到新种群中
model.sol_list.append(copy.deepcopy(f1))
sol_list = copy.deepcopy(model.sol_list) # 先将初始种群进行复制,然后清空,方便填入新个体
model.sol_list = []
while True:
f1_index = int(random.randint(0, len(sol_list) - 1)) # 在种群中随机选择一个索引
f1 = copy.deepcopy(sol_list[f1_index]) # 将索引对应的个体深拷贝
m1_index, m2_index = random.sample(range(len(model.demand_id_list)), 2) # 随机产生个体的两个索引
if m1_index != m2_index:
swap_nodes(f1, m1_index, m2_index)
else:
# 如果相同,则把f1这个个体深拷贝到新种群中
model.sol_list.append(copy.deepcopy(f1))
if len(model.sol_list) > model.popsize:
# 直到新种群中个体数量超过popsize(种群规模)
break
```
可以看到,我们把重复的代码抽象成了内部函数 `swap_nodes`,并使用 `random.sample` 函数来避免重复的代码。代码重构后可读性更好,更加简洁。
阅读全文
相关推荐
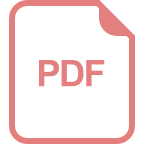
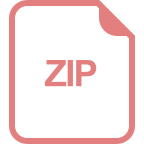














