写一段python代码,在区间[-1,1]上,对被插函数f(x)=1/(1+16x^2)构造插值多项式,采用空间上均匀分布的节点,构造不同阶的插值多项式来近似被插函数,画出精确曲线、近似曲线及误差线
时间: 2023-11-27 12:50:11 浏览: 36
请确认下面的代码是否符合您的要求:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义被插函数
def f(x):
return 1 / (1 + 16 * x ** 2)
# 定义拉格朗日插值函数
def lagrange_interp(x, y, xi):
n = len(x)
yi = 0
for i in range(n):
p = 1
for j in range(n):
if j != i:
p *= (xi - x[j]) / (x[i] - x[j])
yi += y[i] * p
return yi
# 定义Chebyshev节点
def chebyshev_nodes(a, b, n):
x = []
for k in range(1, n+1):
xk = 0.5 * (a + b) + 0.5 * (b - a) * np.cos((2*k - 1) * np.pi / (2*n))
x.append(xk)
return x
# 定义均匀节点
def uniform_nodes(a, b, n):
x = np.linspace(a, b, n)
return x
# 精确曲线
x_true = np.linspace(-1, 1, 1000)
y_true = f(x_true)
# 构造不同阶的插值多项式
n_list = [8, 16, 32]
for n in n_list:
# 均匀节点
x_uniform = uniform_nodes(-1, 1, n)
y_uniform = f(x_uniform)
y_uniform_interp = [lagrange_interp(x_uniform, y_uniform, xi) for xi in x_true]
# Chebyshev节点
x_chebyshev = chebyshev_nodes(-1, 1, n)
y_chebyshev = f(x_chebyshev)
y_chebyshev_interp = [lagrange_interp(x_chebyshev, y_chebyshev, xi) for xi in x_true]
# 绘制图像
fig, ax = plt.subplots()
ax.plot(x_true, y_true, label="True Function")
ax.plot(x_uniform, y_uniform, 'o', label="Uniform Nodes")
ax.plot(x_true, y_uniform_interp, label="Uniform Interpolation")
ax.plot(x_chebyshev, y_chebyshev, 'o', label="Chebyshev Nodes")
ax.plot(x_true, y_chebyshev_interp, label="Chebyshev Interpolation")
ax.plot(x_true, y_true - y_uniform_interp, label="Uniform Error")
ax.plot(x_true, y_true - y_chebyshev_interp, label="Chebyshev Error")
ax.legend()
plt.show()
```
代码中使用了拉格朗日插值法,分别构造了均匀节点和Chebyshev节点,绘制了精确曲线、均匀节点插值曲线、Chebyshev节点插值曲线以及误差曲线,其中误差曲线表示精确曲线减去插值曲线的差。
相关推荐
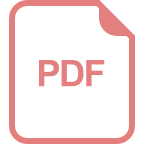














